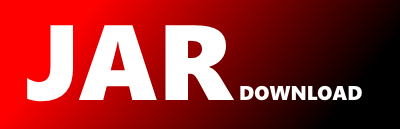
eu.agrosense.api.drmcrop.TimeFrame Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.07.06 at 10:13:00 AM CEST
//
package eu.agrosense.api.drmcrop;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.Duration;
import javax.xml.datatype.XMLGregorianCalendar;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Definition.
* A period of time during which a PropetyValue is measured or is expected to be valid.
*
* Remark
* A measurement can also be made on a particular time, then only StartTime should be given.
*
* DISCUSSION.
* IS TIME FRAME A GOOD EXPRESSION?
*
* The expression Time is used in ISO11783.
* Time is already a defined expression in many computer languages, with a totally different composition.
* Therefore TimeFrame is chosen as expression .
*
* Java class for TimeFrame complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TimeFrame">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="TimeFrameID" type="{http://www.w3.org/2001/XMLSchema}ID"/>
* <element name="StartTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="StopTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="Duration" type="{http://www.w3.org/2001/XMLSchema}duration"/>
* <element name="PropertyValue" type="{http://www.drmCrop.org/schemas/drmCrop}PropertyValue" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TimeFrame", propOrder = {
"timeFrameID",
"startTime",
"stopTime",
"duration",
"propertyValue"
})
public class TimeFrame
implements ToString
{
@XmlElement(name = "TimeFrameID", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String timeFrameID;
@XmlElement(name = "StartTime", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar startTime;
@XmlElement(name = "StopTime", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar stopTime;
@XmlElement(name = "Duration", required = true)
protected Duration duration;
@XmlElement(name = "PropertyValue")
protected List propertyValue;
/**
* Gets the value of the timeFrameID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTimeFrameID() {
return timeFrameID;
}
/**
* Sets the value of the timeFrameID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTimeFrameID(String value) {
this.timeFrameID = value;
}
/**
* Gets the value of the startTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getStartTime() {
return startTime;
}
/**
* Sets the value of the startTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setStartTime(XMLGregorianCalendar value) {
this.startTime = value;
}
/**
* Gets the value of the stopTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getStopTime() {
return stopTime;
}
/**
* Sets the value of the stopTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setStopTime(XMLGregorianCalendar value) {
this.stopTime = value;
}
/**
* Gets the value of the duration property.
*
* @return
* possible object is
* {@link Duration }
*
*/
public Duration getDuration() {
return duration;
}
/**
* Sets the value of the duration property.
*
* @param value
* allowed object is
* {@link Duration }
*
*/
public void setDuration(Duration value) {
this.duration = value;
}
/**
* Gets the value of the propertyValue property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the propertyValue property.
*
*
* For example, to add a new item, do as follows:
*
* getPropertyValue().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PropertyValue }
*
*
*/
public List getPropertyValue() {
if (propertyValue == null) {
propertyValue = new ArrayList();
}
return this.propertyValue;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theTimeFrameID;
theTimeFrameID = this.getTimeFrameID();
strategy.appendField(locator, this, "timeFrameID", buffer, theTimeFrameID);
}
{
XMLGregorianCalendar theStartTime;
theStartTime = this.getStartTime();
strategy.appendField(locator, this, "startTime", buffer, theStartTime);
}
{
XMLGregorianCalendar theStopTime;
theStopTime = this.getStopTime();
strategy.appendField(locator, this, "stopTime", buffer, theStopTime);
}
{
Duration theDuration;
theDuration = this.getDuration();
strategy.appendField(locator, this, "duration", buffer, theDuration);
}
{
List thePropertyValue;
thePropertyValue = (((this.propertyValue!= null)&&(!this.propertyValue.isEmpty()))?this.getPropertyValue():null);
strategy.appendField(locator, this, "propertyValue", buffer, thePropertyValue);
}
return buffer;
}
public TimeFrame withTimeFrameID(String value) {
setTimeFrameID(value);
return this;
}
public TimeFrame withStartTime(XMLGregorianCalendar value) {
setStartTime(value);
return this;
}
public TimeFrame withStopTime(XMLGregorianCalendar value) {
setStopTime(value);
return this;
}
public TimeFrame withDuration(Duration value) {
setDuration(value);
return this;
}
public TimeFrame withPropertyValue(PropertyValue... values) {
if (values!= null) {
for (PropertyValue value: values) {
getPropertyValue().add(value);
}
}
return this;
}
public TimeFrame withPropertyValue(Collection values) {
if (values!= null) {
getPropertyValue().addAll(values);
}
return this;
}
}