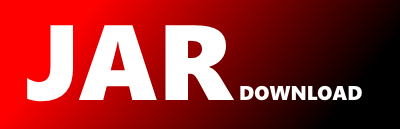
eu.agrosense.client.grid.impl.GridManagerImpl Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*
* Contributors:
* Timon Veenstra - initial API and implementation and/or initial documentation
*/
package eu.agrosense.client.grid.impl;
import com.vividsolutions.jts.geom.Coordinate;
import eu.agrosense.client.grid.Grid;
import eu.agrosense.client.grid.GridCellSize;
import eu.agrosense.client.grid.GridManager;
import eu.agrosense.client.grid.GridProvider;
import eu.agrosense.client.grid.TemporaryGrid;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
import java.util.HashSet;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import nl.cloudfarming.client.farm.model.FarmManager;
import nl.cloudfarming.client.model.AbstractEntityManager;
import nl.cloudfarming.client.model.AgroURI;
import nl.cloudfarming.client.model.EnterpriseIdProvider;
import nl.cloudfarming.client.model.Entity;
import nl.cloudfarming.client.model.ItemIdType;
import org.geotools.geometry.jts.ReferencedEnvelope;
import org.geotools.referencing.crs.DefaultGeographicCRS;
import org.netbeans.api.project.Project;
import org.opengis.geometry.BoundingBox;
import org.openide.filesystems.FileObject;
import org.openide.util.Exceptions;
import org.openide.util.Lookup;
import org.openide.util.lookup.ServiceProvider;
import org.openide.util.lookup.ServiceProviders;
/**
*
* @author Timon Veenstra
*/
@ServiceProviders({
@ServiceProvider(service = GridManager.class),
@ServiceProvider(service = GridProvider.class)
})
public class GridManagerImpl extends AbstractEntityManager implements GridManager, GridProvider {
private static final Logger LOGGER = Logger.getLogger(GridManagerImpl.class.getName());
private static final String GRID_FOLDER_NAME = Grid.ITEM_ID_TYPE.name();
private static final Set SUPPORTED_TYPES = new HashSet<>();
static {
SUPPORTED_TYPES.add(ItemIdType.GRD);
}
@Override
public void save(Grid grid) {
FarmManager farmManager = Lookup.getDefault().lookup(FarmManager.class);
Project project = farmManager.findFarmProject(grid.getURI());
save(grid, project);
}
@Override
public void remove(Grid entity) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public Set getSupportedTypes() {
return SUPPORTED_TYPES;
}
@Override
public boolean supports(ItemIdType itemIdType) {
return ItemIdType.GRD.equals(itemIdType);
}
@Override
public Grid getDefaultGridSection(EnterpriseIdProvider eip, Coordinate topLeft, Coordinate bottomRight) {
return getDefaultGridSection(eip, topLeft, bottomRight, getDefaultGridCellSize());
}
@Override
public Grid getDefaultGridSection(EnterpriseIdProvider eip, Coordinate topLeft, Coordinate bottomRight, GridCellSize cellSize) {
BoundingBox boundingBox = new ReferencedEnvelope(topLeft.x, bottomRight.x, topLeft.y, bottomRight.y, DefaultGeographicCRS.WGS84);
Grid grid = new Grid(eip, cellSize, boundingBox);
return grid;
}
@Override
public GridCellSize getDefaultGridCellSize() {
return GridCellSize.S4;
}
@Override
protected Grid load(AgroURI uri, Project project) {
switch (uri.getItemIdType()) {
case GRD:
FileObject gridObject = getGridFolder(project, true).getFileObject(uri.getItemIdNumber(), Grid.EXT);
return loadGrid(gridObject);
default:
LOGGER.log(Level.WARNING, "cannot load unsupported entity type {0}", new Object[]{uri.getItemIdType()});
return null;
}
}
Grid loadGrid(FileObject fileObject) {
LOGGER.log(Level.FINEST, "loading Grid object from file {0}", fileObject.getName());
assert Grid.EXT.equals(fileObject.getExt()) : "Can only load Grids";
Object object = null;
try (ObjectInputStream ois = new ObjectInputStream(fileObject.getInputStream())) {
object = ois.readObject();
} catch (Exception ex) {
LOGGER.log(Level.SEVERE, "Could not load file {0} with the exception {1}", new Object[]{fileObject, ex});
Exceptions.printStackTrace(ex);
}
assert object instanceof Grid : "fileobject did not contain the right type (Grid)";
Grid grid = (Grid) object;
return getCachedInstanceFor(grid);
}
private FileObject save(Grid grid, Project project) {
LOGGER.log(Level.FINEST, "Saving field with id {0}", new Object[]{grid.getItemIdNumber()});
FileObject gridFolder = getGridFolder(project, true);
FileObject gridObject = gridFolder.getFileObject(grid.getItemIdNumber(), Grid.EXT);
try {
if (gridObject == null) {
String fileName = grid.getItemIdNumber() + "." + Grid.EXT;
// reading by explorer sometimes causes race conditions when using createData():
// "FSException: Cannot get exclusive access to .../path/to/file.ext (probably opened for reading)."
try (OutputStream os = gridFolder.createAndOpen(fileName)) {
save(grid, os, fileName);
}
gridObject = gridFolder.getFileObject(fileName);
} else {
save(grid, gridObject);
}
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not create field file {0} with the exception {1}", new Object[]{grid.getItemIdNumber(), ex});
}
return gridObject;
}
/**
* Get the Grid root Folder
*
* @param project the project to find the folder in
* @param create if the folder should be created if not found
* @return FileObject if found else null
*/
public static FileObject getGridFolder(final Project project, boolean create) {
//Get the stock directory, creating if deleted
FileObject farmFolder = project.getProjectDirectory();
FileObject fieldsFolder = farmFolder.getFileObject(GRID_FOLDER_NAME);
// Create field folder if it doesn't exist
if (fieldsFolder == null && create) {
try {
fieldsFolder = farmFolder.createFolder(GRID_FOLDER_NAME);
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not create the folder {0} in folder {1}", new Object[]{GRID_FOLDER_NAME, farmFolder.getPath()});
}
}
return fieldsFolder;
}
protected void save(Entity entity, OutputStream outputStream, String fileName) {
try (ObjectOutputStream oos = new ObjectOutputStream(outputStream)) {
oos.writeObject(entity);
addToCache(entity);
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not save file {0} with the exception {1}", new Object[]{fileName, ex});
}
}
protected void save(Entity object, FileObject fileObject) {
LOGGER.log(Level.FINEST, "saving {0} object to file {1}", new Object[]{object.getItemIdType(), fileObject.getName()});
// Try-with-resource seems to have slower performance
try (ObjectOutputStream oos = new ObjectOutputStream(fileObject.getOutputStream())) {
oos.writeObject(object);
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not save file {0} with the exception {1}", new Object[]{fileObject, ex});
}
}
@Override
public Grid getTemporaryGridSection(Coordinate topLeft, Coordinate bottomRight) {
return getTemporaryGridSection(topLeft, bottomRight, getDefaultGridCellSize());
}
@Override
public Grid getTemporaryGridSection(Coordinate topLeft, Coordinate bottomRight, GridCellSize cellSize) {
BoundingBox boundingBox = new ReferencedEnvelope(topLeft.x, bottomRight.x, topLeft.y, bottomRight.y, DefaultGeographicCRS.WGS84);
Grid grid = new TemporaryGrid(cellSize, boundingBox);
return grid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy