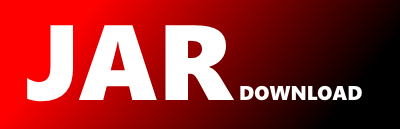
eu.agrosense.client.grid.impl.GridNode Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*
* Contributors:
* Timon Veenstra - initial API and implementation and/or initial documentation
*/
package eu.agrosense.client.grid.impl;
import eu.agrosense.client.grid.Grid;
import java.awt.Image;
import java.awt.datatransfer.Transferable;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.IOException;
import javax.swing.Action;
import org.openide.nodes.FilterNode;
import org.openide.nodes.Node;
import org.openide.nodes.NodeTransfer;
import org.openide.util.ImageUtilities;
import org.openide.util.datatransfer.PasteType;
/**
*
* @author Timon Veenstra
*/
public class GridNode extends FilterNode {
private static final Image ICON = ImageUtilities.loadImage("eu/agrosense/client/grid/grid.png");
private final Grid grid;
public GridNode(Node original, Grid grid) {
super(original, FilterNode.Children.LEAF);
this.grid = grid;
disableDelegation(DELEGATE_SET_NAME);
disableDelegation(DELEGATE_GET_NAME);
updateName();
grid.addPropertyChangeListener(Grid.PROP_NAME, new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
updateName();
}
});
}
private void updateName() {
if (this.grid.getName() != null && !this.grid.getName().isEmpty()) {
setName(this.grid.getName());
setDisplayName(this.grid.getName());
} else {
setName(this.grid.getURI().getItemIdNumber());
setDisplayName(this.grid.getURI().getItemIdNumber());
}
}
@Override
public Action[] getActions(boolean context) {
return super.getActions(context);
// List actions = new ArrayList(Arrays.asList(super.getActions(context)));
// actions.add(new NewActivityFieldAction(field));
// return actions.toArray(new Action[actions.size()]);
}
@Override
public PasteType getDropType(Transferable t, int action, int index) {
// rely on AbstractNode behavior which checks if the transferable contains a NodeTransfer.Paste.
return super.getDropType(t, action, index);
}
@Override
public Transferable clipboardCopy() throws IOException {
return NodeTransfer.transferable(this, NodeTransfer.CLIPBOARD_COPY);
}
@Override
public Transferable clipboardCut() throws IOException {
return clipboardCopy();
}
/**
* This implementation only calls clipboardCopy supposing that copy to
* clipboard and copy by d'n'd are similar.
*
* @return transferable to represent this node during a drag
* @exception IOException when the cut cannot be performed
*/
@Override
public Transferable drag() throws IOException {
return clipboardCopy();
}
@Override
public Image getIcon(int type) {
return ICON;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy