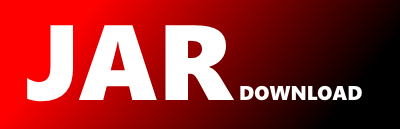
eu.agrosense.client.grid.impl.GridPropertiesElement Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*
* Contributors:
* Timon Veenstra - initial API and implementation and/or initial documentation
*/
package eu.agrosense.client.grid.impl;
import eu.agrosense.client.grid.Grid;
import java.awt.BorderLayout;
import java.io.IOException;
import java.io.InputStream;
import javafx.application.Platform;
import javafx.embed.swing.JFXPanel;
import javafx.fxml.FXMLLoader;
import javafx.fxml.Initializable;
import javafx.fxml.JavaFXBuilderFactory;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.scene.layout.BorderPane;
import javax.swing.Action;
import javax.swing.JComponent;
import javax.swing.JPanel;
import javax.swing.JToolBar;
import org.netbeans.core.spi.multiview.CloseOperationState;
import org.netbeans.core.spi.multiview.MultiViewElement;
import org.netbeans.core.spi.multiview.MultiViewElementCallback;
import org.openide.awt.UndoRedo;
import org.openide.util.Exceptions;
import org.openide.util.Lookup;
import org.openide.util.NbBundle;
import org.openide.windows.TopComponent;
/**
*
* @author Timon Veenstra
*/
@MultiViewElement.Registration(
displayName = "#LBL_Grid_PROPERTIES",
iconBase = "eu/agrosense/client/grid/impl/grid.png",
mimeType = "application/x-agrosense-grid",
persistenceType = TopComponent.PERSISTENCE_NEVER,
preferredID = "GridProperties",
position = 3000)
@NbBundle.Messages("LBL_Grid_PROPERTIES=Properties")
public class GridPropertiesElement extends JPanel implements MultiViewElement {
protected GridDataObject gridDataObject;
private JToolBar toolbar = new JToolBar();
private transient MultiViewElementCallback callback;
private JFXPanel fxPanel;
private GridPropertiesController controller;
public GridPropertiesElement(Lookup lkp) {
gridDataObject = lkp.lookup(GridDataObject.class);
assert gridDataObject != null;
setLayout(new BorderLayout());
initComponents();
}
@Override
public String getName() {
return "GridPropertiesElement";
}
private void initComponents() {
setLayout(new BorderLayout());
fxPanel = new JFXPanel();
// calendarDropTarget = new CalendarDropTarget();
Platform.setImplicitExit(false);
// create JavaFX scene
Platform.runLater(new Runnable() {
public void run() {
// Parent root;
try {
// root = FXMLLoader.load(getClass().getResource("/eu/agrosense/client/grid/impl/GridPropertiesElement.fxml"));
// Scene scene = new Scene(root);
// fxPanel.setScene(scene);
String fxml = "GridPropertiesElement.fxml";
FXMLLoader loader = new FXMLLoader();
InputStream in = GridPropertiesElement.class.getResourceAsStream(fxml);
loader.setBuilderFactory(new JavaFXBuilderFactory());
loader.setLocation(GridPropertiesElement.class.getResource(fxml));
AnchorPane page;
try {
page = (AnchorPane) loader.load(in);
} finally {
in.close();
}
Scene scene = new Scene(page, 800, 600);
fxPanel.setScene(scene);
controller = loader.getController();
controller.setGrid(gridDataObject.getLookup().lookup(Grid.class));
} catch (IOException ex) {
Exceptions.printStackTrace(ex);
}
}
});
// fxPanel.setDropTarget(calendarDropTarget);
add(fxPanel, BorderLayout.CENTER);
}
// protected void initFX(JFXPanel fxPanel) {
// Scene scene = createScene();
// fxPanel.setScene(scene);
// }
// private Scene createScene() {
// BorderPane borderPane = new BorderPane();
// Scene scene = new Scene(borderPane);
//
// scene.getStylesheets().add(GridPropertiesElement.class.getResource("GridPropertiesElement.css").toExternalForm());
//// borderPane.setCenter(calendarComponent);
// return scene;
// }
@Override
public JComponent getVisualRepresentation() {
return this;
}
@Override
public JComponent getToolbarRepresentation() {
return toolbar;
}
@Override
public Action[] getActions() {
return new Action[0];
}
@Override
public Lookup getLookup() {
return gridDataObject.getLookup();
}
@Override
public void componentOpened() {
}
@Override
public void componentClosed() {
}
@Override
public void componentShowing() {
if (callback != null) {
updateName();
}
}
@Override
public void componentHidden() {
}
@Override
public void componentActivated() {
}
@Override
public void componentDeactivated() {
}
@Override
public UndoRedo getUndoRedo() {
return UndoRedo.NONE;
}
@Override
public void setMultiViewCallback(MultiViewElementCallback callback) {
this.callback = callback;
}
@Override
public CloseOperationState canCloseElement() {
return CloseOperationState.STATE_OK;
}
private void updateName() {
if (callback != null) {
Grid grid = gridDataObject.getLookup().lookup(Grid.class);
TopComponent tc = callback.getTopComponent();
tc.setHtmlDisplayName(grid.getName());
tc.setDisplayName(grid.getName());
tc.setName(getName());
tc.setToolTipText(getToolTipText());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy