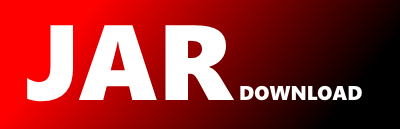
eu.agrosense.client.grid.impl.GridsNode Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*
* Contributors:
* Timon Veenstra - initial API and implementation and/or initial documentation
*/
package eu.agrosense.client.grid.impl;
import eu.agrosense.client.grid.Grid;
import eu.agrosense.client.grid.GridManager;
import eu.agrosense.client.grid.TemporaryGrid;
import java.awt.Image;
import java.awt.datatransfer.Transferable;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.Action;
import nl.cloudfarming.client.field.model.ShapeCollection;
import nl.cloudfarming.client.geoviewer.Palette;
import nl.cloudfarming.client.geoviewer.RandomColorPalette;
import nl.cloudfarming.client.geoviewer.RangedLegendPalette;
import nl.cloudfarming.client.model.EnterpriseIdProvider;
import nl.cloudfarming.client.util.UOM;
import org.netbeans.api.project.Project;
import org.openide.filesystems.FileObject;
import org.openide.nodes.FilterNode;
import org.openide.nodes.Node;
import org.openide.nodes.NodeTransfer;
import org.openide.util.ImageUtilities;
import org.openide.util.Lookup;
import org.openide.util.NbBundle;
import org.openide.util.datatransfer.PasteType;
/**
*
* @author Timon Veenstra
*/
@NbBundle.Messages("Grids node name=Grids")
final class GridsNode extends FilterNode {
private final Project project;
private static final Image ICON = ImageUtilities.loadImage("eu/agrosense/client/grid/grid.png");
private static final Logger LOGGER = Logger.getLogger(GridsNode.class.getName());
public GridsNode(Node original, Project project) {
super(original, new GridChildren(original), original.getLookup());
this.project = project;
disableDelegation(DELEGATE_SET_NAME);
setName(Bundle.Grids_node_name());
setDisplayName(Bundle.Grids_node_name());
}
@Override
public Image getIcon(int type) {
return ICON;
}
@Override
public Image getOpenedIcon(int type) {
return getIcon(type);
}
@Override
public boolean canCopy() {
return false;
}
@Override
public boolean canRename() {
return true;
}
@Override
public boolean canCut() {
return false;
}
@Override
public PasteType getDropType(final Transferable transferable, int action, int index) {
final GridManager gridManager = Lookup.getDefault().lookup(GridManager.class);
final Node[] nodes = NodeTransfer.nodes(transferable, NodeTransfer.DND_COPY);
if (nodes != null && acceptNode(nodes) && gridManager != null) {
return new PasteType() {
@Override
public Transferable paste() throws IOException {
processDroppedNodes(nodes, gridManager);
return null;
}
};
}
return null;
}
/**
*
* @param nodes
* @param gridManager
*/
private void processDroppedNodes(Node[] nodes, final GridManager gridManager) {
LOGGER.log(Level.FINEST, "incoming {0} dropped nodes", nodes.length);
for (Node node : nodes) {
processDroppedNode(node, gridManager);
}
}
private void processDroppedNode(Node node, final GridManager gridManager) {
LOGGER.log(Level.FINEST, "processing dropped node {0}", node);
Grid droppedGrid = node.getLookup().lookup(Grid.class);
if (droppedGrid != null) {
if (droppedGrid instanceof TemporaryGrid) {
EnterpriseIdProvider eip = project.getLookup().lookup(EnterpriseIdProvider.class);
Grid grid = new Grid(eip, droppedGrid);
gridManager.save(grid);
} else {
gridManager.save(droppedGrid);
}
}
}
@Override
public Transferable drag() throws IOException {
return clipboardCopy();
}
@Override
public Transferable clipboardCopy() throws IOException {
return NodeTransfer.transferable(this, NodeTransfer.CLIPBOARD_COPY);
}
@Override
public Action[] getActions(boolean context) {
//TODO add new grid action
return new Action[]{};
}
/**
* Helper method to ease the process of accepting multiple nodes. This
* method calls acceptNode for each single node of a passed node array and
* validates the node.
*
* @param nodes Array of nodes that should be tested for acceptance
* @return false if any of the nodes in the passed array does not contain a
* lookup with a {@link ShapeCollection}, otherwise true
*/
protected boolean acceptNode(Node[] nodes) {
for (Node node : nodes) {
if (!acceptNode(node)) {
return false;
}
}
return true;
}
protected boolean acceptNode(Node node) {
return node.getLookup().lookup(Grid.class)!=null;
}
private static class GridChildren extends FilterNode.Children {
public GridChildren(Node or) {
super(or);
}
@Override
protected Node[] createNodes(Node key) {
List result = new ArrayList<>();
for (Node node : super.createNodes(key)) {
if (accept(node)) {
result.add(node);
// result.add(node);
}
}
return result.toArray(new Node[result.size()]);
}
// only accept non fileobject nodes or nodes that do not have a
// folder fileobject in their lookup
private boolean accept(Node node) {
return node.getLookup().lookup(FileObject.class) == null
|| !node.getLookup().lookup(FileObject.class).isFolder();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy