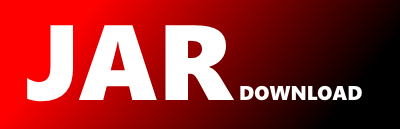
eu.agrosense.client.io.csv.CsvFileAttributes Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package eu.agrosense.client.io.csv;
import java.io.Serializable;
import java.util.Objects;
import org.openide.util.NbBundle;
/**
*
* @author johan
*/
public class CsvFileAttributes implements Serializable {
private char separatorChar;
private Character quoteChar;
private Character escapeChar;
private int skipLines;
public CsvFileAttributes(char separatorChar, char quoteChar, char escapeChar, int skipLines) {
this.separatorChar = separatorChar;
this.quoteChar = quoteChar;
this.escapeChar = escapeChar;
this.skipLines = skipLines;
}
public CsvFileAttributes(char separatorChar, int skipLines) {
this(separatorChar, CsvDataObject.DEFAULT_QUOTE_CHAR, CsvDataObject.DEFAULT_ESCAPE_CHAR, skipLines);
}
public CsvFileAttributes(char separatorChar) {
this(separatorChar, CsvDataObject.DEFAULT_SKIP_LINES);
}
public char getSeparatorChar() {
return separatorChar;
}
public Character getQuoteChar() {
return quoteChar;
}
public Character getEscapeChar() {
return escapeChar;
}
public int getSkipLines() {
return skipLines;
}
@Override
public int hashCode() {
int hash = 7;
hash = 53 * hash + this.separatorChar;
hash = 53 * hash + Objects.hashCode(this.quoteChar);
hash = 53 * hash + Objects.hashCode(this.escapeChar);
hash = 53 * hash + this.skipLines;
return hash;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final CsvFileAttributes other = (CsvFileAttributes) obj;
if (this.separatorChar != other.separatorChar) {
return false;
}
if (!Objects.equals(this.quoteChar, other.quoteChar)) {
return false;
}
if (!Objects.equals(this.escapeChar, other.escapeChar)) {
return false;
}
if (this.skipLines != other.skipLines) {
return false;
}
return true;
}
private static interface CharacterAttribute {
Character getCharacter(); // allow nulls for quote/escape char
String getDescription();
}
private static T find(T[] cas, Character c) {
for (T ca : cas) {
if (Objects.equals(c, ca.getCharacter())) { return ca; }
}
return null;
}
@NbBundle.Messages({
"CsvFileAttributes.Separator.COMMA=Comma",
"CsvFileAttributes.Separator.SEMICOLON=Semicolon",
"CsvFileAttributes.Separator.TAB=Tab",
"CsvFileAttributes.Separator.SPACE=Space",
"CsvFileAttributes.Separator.COLON=Colon",
"CsvFileAttributes.Separator.PIPE=Pipe",
})
public static enum Separator implements CharacterAttribute {
COMMA(','),
SEMICOLON(';'),
TAB('\t'),
SPACE(' '),
COLON(':'),
PIPE('|'),
;
private final Character character;
private Separator(Character character) {
this.character = character;
}
@Override
public Character getCharacter() {
return character;
}
@Override
public String getDescription() {
return NbBundle.getMessage(CsvFileAttributes.class, "CsvFileAttributes.Separator." + name());
}
@Override
public String toString() {
return getDescription();
}
public static Separator find(Character c) {
return CsvFileAttributes.find(Separator.values(), c);
}
}
@NbBundle.Messages({
"CsvFileAttributes.Quote.NONE=None",
"CsvFileAttributes.Quote.DOUBLE_QUOTE=Double quotation mark",
"CsvFileAttributes.Quote.SINGLE_QUOTE=Single quotation mark",
})
public static enum Quote implements CharacterAttribute {
NONE(null),
DOUBLE_QUOTE('"'),
SINGLE_QUOTE('\''),
;
private final Character character;
private Quote(Character character) {
this.character = character;
}
@Override
public Character getCharacter() {
return character;
}
@Override
public String getDescription() {
return NbBundle.getMessage(CsvFileAttributes.class, "CsvFileAttributes.Quote." + name());
}
@Override
public String toString() {
return getDescription();
}
public static Quote find(Character c) {
return CsvFileAttributes.find(Quote.values(), c);
}
}
@NbBundle.Messages({
"CsvFileAttributes.Escape.NONE=None",
"CsvFileAttributes.Escape.BACKSLASH=Backslash",
})
public static enum Escape implements CharacterAttribute {
NONE(null),
BACKSLASH('\\'),
;
private final Character character;
private Escape(Character character) {
this.character = character;
}
@Override
public Character getCharacter() {
return character;
}
@Override
public String getDescription() {
return NbBundle.getMessage(CsvFileAttributes.class, "CsvFileAttributes.ESCAPE." + name());
}
@Override
public String toString() {
return getDescription();
}
public static Escape find(Character c) {
return CsvFileAttributes.find(Escape.values(), c);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy