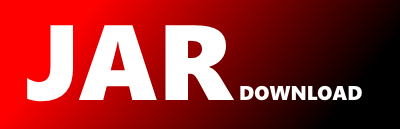
eu.agrosense.client.lib.geotools.UTMZone Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2011 Agrosense
*
* Licensed under the Eclipse Public License - v 1.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.agrosense.client.lib.geotools;
/**
*
* Represents an UTMZone.
*
* UTM is a world covering projection, where each hemisphere is divided into
* 60 parts. This provides a global working projection method for area
* calculations.
*
* To retrieve the UTMZone for a geometry, use {@link UTMZone#fromGPSCoordinates(double, double) }
* and provide the coordinate from the center point. Even if the geometry
* would be on the border of 2 zones, both zones would be just as accurate,
* so the center point is a valid option.
*
*
* @author Timon Veenstra
*/
public class UTMZone {
public enum Hemisphere{
NORTHERN, SOUTHERN
}
private final Hemisphere hemisphere;
private final int number;
private final String EPSG;
public UTMZone( int number,Hemisphere hemisphere) {
this.hemisphere = hemisphere;
this.number = number;
if (number<0 || number>60){
throw new IllegalArgumentException("Argument number should be between 0 and 60");
}
String no = String.valueOf(number);
no = (no.length()==1)?"0"+no:no;
EPSG = "EPSG:32"+(Hemisphere.NORTHERN.equals(hemisphere)?"6":"7")+no;
}
public String getEPSG() {
return EPSG;
}
public Hemisphere getHemisphere() {
return hemisphere;
}
public int getNumber() {
return number;
}
/**
* Determine the UTMZone for given latitude and longitude.
*
* @param latitude
* @param longitude
* @return
*/
public static UTMZone fromGPSCoordinates(double latitude, double longitude){
return new UTMZone(longitudeToZoneNumber(longitude), latitudeToHemisphere(latitude));
}
public static int longitudeToZoneNumber(double longitude){
return new Double(Math.floor((longitude+180.0)/6.0)).intValue()+1;
}
public static Hemisphere latitudeToHemisphere(double latitude){
return (latitude >= 0)?Hemisphere.NORTHERN:Hemisphere.SOUTHERN;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final UTMZone other = (UTMZone) obj;
if (this.hemisphere != other.hemisphere) {
return false;
}
if (this.number != other.number) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 7;
hash = 61 * hash + (this.hemisphere != null ? this.hemisphere.hashCode() : 0);
hash = 61 * hash + this.number;
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy