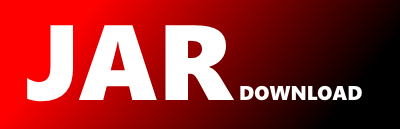
eu.agrosense.spi.session.SessionController Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.agrosense.spi.session;
import eu.agrosense.api.session.AuthorizationMethod;
import eu.agrosense.api.session.AuthorizationCallback;
import eu.agrosense.api.session.OnFailureAction;
import eu.agrosense.api.session.Session;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Timer;
import java.util.TimerTask;
import javafx.application.Platform;
import org.opendolphin.core.PresentationModel;
import org.openide.util.Lookup;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* There is only one client-server session. Although there might be several
* connection from the client to the server (1 root and 1 per farm), they all
* share the same UserSession presentation model object.
*
* The farm sessions are controlled by the {@link FarmSessionController }
*
* @author Timon Veenstra
*/
public class SessionController {
private static final Logger log = LoggerFactory.getLogger(SessionController.class);
private static final SessionController INSTANCE = new SessionController();
private final SessionImpl session = new SessionImpl();
private static final int TIMEOUT_SECONDS = 30;
static SessionController getInstance() {
return INSTANCE;
}
private SessionController() {
}
Session getSession() {
return this.session;
}
PresentationModel getSessionModel() {
return this.session.getModel();
}
void validateSession(final AuthorizationCallback authorizationCallback) {
// this is save place to check for timeout on server handshake
if (session.isServerHandShook()) {
if (session.isUserLoginRequired()) {
presentLogin(authorizationCallback);
}
//TODO catch strange situations like when user login is not required but session also not valid
} else {
// wait for server handshake
session.addServerHandShakeListener(new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
if ((boolean) evt.getNewValue()) {
validateSession(authorizationCallback);
}
}
});
// add timer thread for connection
Timer connectionCheckerTimer = new Timer(true);
connectionCheckerTimer.scheduleAtFixedRate(
new TimerTask() {
public void run() {
if (!session.isServerHandShook()) {
Platform.runLater(new Runnable() {
@Override
public void run() {
log.debug("triggering server timeout");
triggerServerTimeout();
}
});
}
}
}, 0, TIMEOUT_SECONDS * 1000);
}
}
private void triggerServerTimeout() {
for (AuthorizationMethod method : Lookup.getDefault().lookupAll(AuthorizationMethod.class)) {
method.triggerFailure(OnFailureAction.Type.SERVER_TIMEOUT);
}
}
private void presentLogin(final AuthorizationCallback authorizationCallback) {
for (AuthorizationMethod method : Lookup.getDefault().lookupAll(AuthorizationMethod.class)) {
if (session.getMethod().equals(method.getSupportedMethod())) {
method.authorize(authorizationCallback, session.getModel());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy