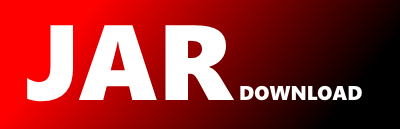
eu.binjr.common.function.CheckedLambdas Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binjr-core Show documentation
Show all versions of binjr-core Show documentation
A Time Series Data Browser
/*
* Copyright 2017-2018 Frederic Thevenet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.binjr.common.function;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* A series of methods that wrap functional interfaces that throw checked
* exceptions inside their standard (non throwing) counterparts, so they can be
* used with streams or other classes expecting standards functional interfaces
* and have them acting transparent to the exception thrown within the lambda.
*
* @author Frederic Thevenet
*/
public final class CheckedLambdas {
/**
* Override constructor
*/
private CheckedLambdas() {
}
/**
* Wraps a {@link CheckedConsumer} inside a {@link Consumer} and rethrow the
* original exception.
*
* @param consumer the {@link Consumer} to wrap.
* @param the type for the consumer's parameter.
* @param the type for the checked exception.
* @return a {@link Consumer} instance.
* @throws E the checked exception thrown in the lambda.
*/
public static Consumer wrap(CheckedConsumer consumer) throws E {
return t -> {
try {
consumer.accept(t);
} catch (Exception exception) {
throw throwActualException(exception);
}
};
}
// public static Predicate wrap(CheckedPredicate predicate) throws E {
// return t -> {
// try {
// return predicate.test(t);
// } catch (Exception exception) {
// throw throwActualException(exception);
// }
// };
// }
/**
* Wraps a {@link CheckedRunnable} inside a {@link Runnable} and rethrow the
* original exception.
*
* @param runnable the {@link Runnable} to wrap.
* @param the type for the checked exception.
* @return a {@link Runnable} instance.
* @throws E the checked exception thrown in the lambda.
*/
public static Runnable wrap(CheckedRunnable runnable) throws E {
return () -> {
try {
runnable.run();
} catch (Exception exception) {
throw throwActualException(exception);
}
};
}
/**
* Wraps a {@link CheckedSupplier} inside a {@link Supplier} and rethrow the
* original exception.
*
* @param supplier the {@link Supplier} to wrap.
* @param the type for the supplier's parameter.
* @param the type for the checked exception.
* @return a {@link Supplier} instance.
* @throws E the checked exception thrown in the lambda.
*/
public static Supplier wrap(CheckedSupplier supplier) throws E {
return () -> {
try {
return supplier.get();
} catch (Exception exception) {
throw throwActualException(exception);
}
};
}
/**
* Wraps a {@link CheckedFunction} inside a {@link Function} and rethrow the
* original exception.
*
* @param function the {@link Function} to wrap.
* @param the type for the function's parameter.
* @param the return type of the function.
* @param the type for the checked exception.
* @return a {@link Function} instance.
* @throws E the checked exception thrown in the lambda.
*/
public static Function wrap(CheckedFunction function) throws E {
return t -> {
try {
return function.apply(t);
} catch (Exception exception) {
throw throwActualException(exception);
}
};
}
/**
* Forge runtime exception
*
* @param exception exception
* @return Runtime exception
* @throws E Exception
*/
@SuppressWarnings("unchecked")
private static RuntimeException throwActualException(Exception exception) throws E {
throw (E) exception;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy