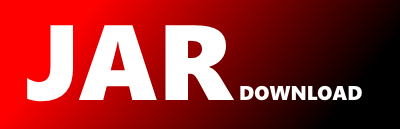
eu.binjr.common.javafx.controls.ToolButtonBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binjr-core Show documentation
Show all versions of binjr-core Show documentation
A Time Series Data Browser
/*
* Copyright 2019-2021 Frederic Thevenet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.binjr.common.javafx.controls;
import eu.binjr.common.javafx.bindings.BindingManager;
import eu.binjr.core.preferences.UserPreferences;
import javafx.beans.binding.Bindings;
import javafx.beans.property.Property;
import javafx.beans.value.ObservableValue;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.control.ButtonBase;
import javafx.scene.control.ContentDisplay;
import javafx.scene.control.Tooltip;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Region;
import javafx.util.Duration;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
public class ToolButtonBuilder {
private final BindingManager bindingManager;
private double height = 20.0;
private double width = 20.0;
private String text = "";
private String tooltip = "";
private List styleClass = new ArrayList<>();
private List iconStyleClass = new ArrayList<>();
private EventHandler action = null;
private final List> bindings = new ArrayList<>();
private boolean focusTraversable = true;
public ToolButtonBuilder() {
this(null);
}
public ToolButtonBuilder(BindingManager bindingManager) {
this.bindingManager = bindingManager;
}
private T buildButton(T btn) {
btn.setText(text);
btn.setTooltip(makeTooltip(tooltip));
btn.setPrefHeight(height);
btn.setMaxHeight(height);
btn.setMinHeight(height);
btn.setPrefWidth(width);
btn.setMaxWidth(width);
btn.setMinWidth(width);
btn.setFocusTraversable(focusTraversable);
if (styleClass != null) {
btn.getStyleClass().addAll(styleClass);
}
if (iconStyleClass != null && !iconStyleClass.isEmpty()) {
Region icon = new Region();
icon.getStyleClass().addAll(iconStyleClass);
btn.setGraphic(icon);
btn.setAlignment(Pos.CENTER);
btn.setContentDisplay(ContentDisplay.GRAPHIC_ONLY);
} else {
btn.setContentDisplay(ContentDisplay.TEXT_ONLY);
}
if (action != null) {
btn.setOnAction(bindingManager != null ? bindingManager.registerHandler(action) : action);
}
bindings.forEach(buttonBaseConsumer -> buttonBaseConsumer.accept(btn));
return btn;
}
public ToolButtonBuilder setHeight(double height) {
this.height = height;
return this;
}
public ToolButtonBuilder setWidth(double width) {
this.width = width;
return this;
}
public ToolButtonBuilder setFocusTraversable(boolean focusTraversable){
this.focusTraversable = focusTraversable;
return this;
}
public ToolButtonBuilder setText(String text) {
this.text = text;
return this;
}
public ToolButtonBuilder setTooltip(String tooltip) {
this.tooltip = tooltip;
return this;
}
public ToolButtonBuilder setStyleClass(String... styleClass) {
this.styleClass = new ArrayList<>(Arrays.asList(styleClass));
return this;
}
public ToolButtonBuilder setIconStyleClass(String... iconStyleClass) {
this.iconStyleClass = new ArrayList<>(Arrays.asList(iconStyleClass));
return this;
}
public ToolButtonBuilder setAction(EventHandler action) {
this.action = action;
return this;
}
public ToolButtonBuilder bindBidirectionnal(Function> mapper, Property property) {
bindings.add(t -> {
if (bindingManager != null) {
bindingManager.bindBidirectional(mapper.apply(t), property);
} else {
mapper.apply(t).bindBidirectional(property);
}
});
return this;
}
public ToolButtonBuilder bind(Function> mapper, ObservableValue observableValue) {
bindings.add(buttonBase -> {
if (bindingManager != null) {
bindingManager.bind(mapper.apply(buttonBase), observableValue);
} else {
mapper.apply(buttonBase).bind(observableValue);
}
});
return this;
}
public T build(Supplier generator) {
return buildButton(generator.get());
}
public static Node makeIconNode(Pos position, String... iconStyles) {
Region r = new Region();
r.getStyleClass().addAll(iconStyles);
HBox box = new HBox(r);
box.setAlignment(position);
box.getStyleClass().add("icon-container");
return box;
}
private Tooltip makeTooltip(String text) {
var tooltip = new Tooltip(text);
var delayProp = UserPreferences.getInstance().tooltipShowDelayMs.property();
tooltip.showDelayProperty().bind(Bindings.createObjectBinding(() -> Duration.millis(delayProp.getValue().doubleValue()), delayProp));
return tooltip;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy