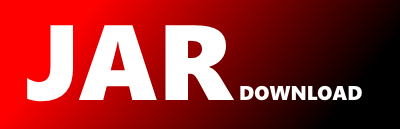
eu.cedarsoft.utils.StringCmdLine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cmd Show documentation
Show all versions of cmd Show documentation
Utils for access to the command line.
The newest version!
package eu.cedarsoft.utils;
import org.jetbrains.annotations.NonNls;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Command Line implementation that is based on strings.
*
* Date: 25.09.2006
* Time: 19:43:04
*
* @author Johannes Schneider -
* Xore Systems
*/
public class StringCmdLine extends AbstractCmdLine implements CmdLine {
private int expectedIndex;
private List expectedOut = new ArrayList();
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy