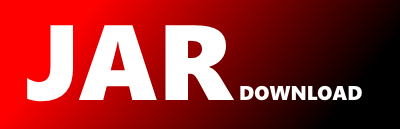
eu.ciechanowiec.sling.rocket.asset.NTResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sling.rocket.commons Show documentation
Show all versions of sling.rocket.commons Show documentation
Common utilities used by Sling Rocket
package eu.ciechanowiec.sling.rocket.asset;
import eu.ciechanowiec.sling.rocket.commons.ResourceAccess;
import eu.ciechanowiec.sling.rocket.jcr.NTFile;
import eu.ciechanowiec.sling.rocket.jcr.NodeProperties;
import eu.ciechanowiec.sling.rocket.jcr.path.JCRPath;
import eu.ciechanowiec.sling.rocket.jcr.path.ParentJCRPath;
import eu.ciechanowiec.sling.rocket.jcr.path.TargetJCRPath;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
import org.apache.jackrabbit.JcrConstants;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import java.util.Optional;
@Slf4j
@ToString
class NTResource implements Asset {
@SuppressWarnings("PMD.AvoidFieldNameMatchingMethodName")
private final JCRPath jcrPath;
@ToString.Exclude
private final ResourceAccess resourceAccess;
NTResource(Resource resource, ResourceAccess resourceAccess) {
String resourcePath = resource.getPath();
this.jcrPath = new TargetJCRPath(resourcePath);
this.resourceAccess = resourceAccess;
assertPrimaryType();
assertParentNodeType();
log.trace("Initialized {}", this);
}
private void assertPrimaryType() {
log.trace("Asserting primary type of {}", this);
NodeProperties nodeProperties = new NodeProperties(jcrPath, resourceAccess);
nodeProperties.assertPrimaryType(JcrConstants.NT_RESOURCE);
}
private void assertParentNodeType() {
ParentJCRPath parentJCRPath = parentPath();
log.trace("Asserting primary type of {}", parentJCRPath);
NodeProperties parentNP = new NodeProperties(parentJCRPath, resourceAccess);
parentNP.assertPrimaryType(JcrConstants.NT_FILE);
}
private ParentJCRPath parentPath() {
return new JCRPathWithParent(jcrPath, resourceAccess).parent().orElseThrow();
}
private Asset parentAsset() {
ParentJCRPath parentJCRPath = parentPath();
log.trace("Parent JCR path for {} is {}", this, parentJCRPath);
try (ResourceResolver resourceResolver = resourceAccess.acquireAccess()) {
String parentJCRPathRaw = parentJCRPath.get();
return Optional.ofNullable(resourceResolver.getResource(parentJCRPathRaw))
.map(resource -> new NTFile(resource, resourceAccess))
.orElseThrow();
}
}
@Override
public AssetFile assetFile() {
return parentAsset().assetFile();
}
@Override
public AssetMetadata assetMetadata() {
return parentAsset().assetMetadata();
}
@Override
public String jcrUUID() {
return parentAsset().jcrUUID();
}
@Override
public JCRPath jcrPath() {
return parentAsset().jcrPath();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy