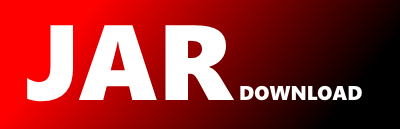
eu.ciechanowiec.sling.rocket.commons.UnwrappedIteration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sling.rocket.commons Show documentation
Show all versions of sling.rocket.commons Show documentation
Common utilities used by Sling Rocket
package eu.ciechanowiec.sling.rocket.commons;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Spliterator;
import java.util.concurrent.CompletableFuture;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* Data structure operations on the wrapped {@link Iterator} and {@link Iterable}.
*
* @param the type of elements in the wrapped data structure
*/
@SuppressWarnings("WeakerAccess")
public class UnwrappedIteration {
private final CompletableFuture> unwrappedCollection;
/**
* Constructs an instance of this class.
* @param iterator {@link Iterator} to be unwrapped
*/
public UnwrappedIteration(Iterator iterator) {
unwrappedCollection = CompletableFuture.supplyAsync(() -> asCollection(iterator));
}
/**
* Constructs an instance of this class.
* @param iterable {@link Iterable} to be unwrapped
*/
public UnwrappedIteration(Iterable iterable) {
unwrappedCollection = CompletableFuture.supplyAsync(() -> asCollection(iterable));
}
private Collection asCollection(Iterator iterator) {
return unwrap(iterator).toList();
}
private Collection asCollection(Iterable iterable) {
return unwrap(iterable).toList();
}
private Stream unwrap(Iterator iterator) {
Iterable iterable = () -> iterator;
return unwrap(iterable);
}
private Stream unwrap(Iterable iterable) {
Spliterator spliterator = iterable.spliterator();
return StreamSupport.stream(spliterator, false);
}
/**
* Returns a {@link Stream} of elements from the wrapped data structure.
*
* @return {@link Stream} of elements from the wrapped data structure
*/
public Stream stream() {
Collection collection = unwrappedCollection.join();
return collection.stream();
}
/**
* Returns an unmodifiable {@link List} of elements from the wrapped data structure.
* A new object is returned each time this method is called.
*
* @return unmodifiable {@link List} of elements from the wrapped data structure
*/
public List list() {
return stream().toList();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy