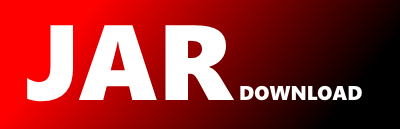
eu.ciechanowiec.sling.rocket.llm.ChatMessageDefault Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sling.rocket.commons Show documentation
Show all versions of sling.rocket.commons Show documentation
Common utilities used by Sling Rocket
package eu.ciechanowiec.sling.rocket.llm;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
import java.util.function.Supplier;
import java.util.function.UnaryOperator;
/**
* Default implementation of {@link ChatMessage}.
*/
@SuppressWarnings("WeakerAccess")
@ToString
@Slf4j
public class ChatMessageDefault implements ChatMessage {
@SuppressWarnings("PMD.AvoidFieldNameMatchingMethodName")
@ToString.Exclude
private final Supplier roleSupplier;
@ToString.Exclude
private final Supplier contentSupplier;
/**
* Constructs an instance of this class.
* @param role type of the author of this {@link ChatMessage}
* @param content content of this {@link ChatMessage}
*/
@JsonCreator
public ChatMessageDefault(@JsonProperty("role") Role role, @JsonProperty("content") String content) {
this.roleSupplier = () -> role;
this.contentSupplier = () -> content;
log.trace("Initialized {} with content: '{}'", this, content);
}
/**
* Constructs an instance of this class.
* @param roleSupplier {@link Supplier} that produces the type of the author of this {@link ChatMessage}
* @param contentSupplier {@link Supplier} that produces the content of this {@link ChatMessage}
*/
public ChatMessageDefault(Supplier roleSupplier, Supplier contentSupplier) {
this.roleSupplier = roleSupplier;
this.contentSupplier = contentSupplier;
log.trace("Initialized {}", this);
}
/**
* Constructs an instance of this class.
* @param sourceMessage {@link ChatMessage} to be used as a source of the {@link Role}
* and content for the newly constructed {@link ChatMessage}
* @param contentTransformer function that will be applied to the content of the {@code sourceMessage}
* and to produce the content of the newly constructed {@link ChatMessage}
*/
public ChatMessageDefault(ChatMessage sourceMessage, UnaryOperator contentTransformer) {
this.roleSupplier = sourceMessage::role;
String initialContent = sourceMessage.content();
this.contentSupplier = () -> contentTransformer.apply(initialContent);
log.trace("Initialized {}. Initial content: '{}'", this, initialContent);
}
/**
* {@inheritDoc}
*/
@Override
@JsonProperty("content")
public String content() {
return contentSupplier.get();
}
/**
* {@inheritDoc}
*/
@Override
@JsonProperty("role")
public Role role() {
return roleSupplier.get();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy