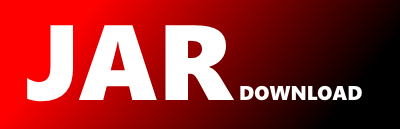
eu.ciechanowiec.sling.rocket.asset.AssetMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sling.rocket.commons Show documentation
Show all versions of sling.rocket.commons Show documentation
Common utilities used by Sling Rocket
package eu.ciechanowiec.sling.rocket.asset;
import eu.ciechanowiec.conditional.Conditional;
import eu.ciechanowiec.sling.rocket.jcr.NodeProperties;
import org.apache.tika.mime.MimeType;
import org.apache.tika.mime.MimeTypeException;
import org.apache.tika.mime.MimeTypes;
import javax.jcr.Node;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
/**
* Represents metadata of an {@link Asset}, either existing or a future one. Might be of two types:
*
* - Backed by a persistent {@link Node} of type {@link Asset#NT_ASSET_METADATA}.
* - Backed by an in-memory data structure.
*
*/
public interface AssetMetadata {
/**
* Name of the mime type property of the associated {@link Asset}.
*/
String PN_MIME_TYPE = "mimeType";
/**
* Returns the mime type of the associated {@link Asset}.
* @return mime type of the associated {@link Asset}
*/
String mimeType();
/**
* Returns all properties of the associated {@link Asset} as a {@link Map}
* of property names to property values converted to {@link String}; if a
* given value cannot be converted to {@link String}, it is omitted from the result.
* @return all properties of the associated {@link Asset} as a {@link Map}
* of property names to property values converted to {@link String}; if a
* given value cannot be converted to {@link String}, it is omitted from the result
*/
Map all();
/**
* Returns a {@link Map} of all properties returned by {@link AssetMetadata#all()},
* where all values are cast to {@link Object}-s.
* @return {@link Map} of all properties returned by {@link AssetMetadata#all()}, where
* all values are cast to {@link Object}-s
*/
@SuppressWarnings("unchecked")
default Map allButObjectValues() {
return (Map) (Map, ?>) all();
}
/**
* Returns an {@link Optional} containing the filename extension of the associated {@link Asset}
* and prepended with a dot, e.g. {@code '.txt'}, {@code '.jpg'}, {@code '.pdf'};
* if the filename extension cannot be determined, an empty {@link Optional} is returned.
* @return {@link Optional} containing the filename extension of the associated {@link Asset}
* and prepended with a dot, e.g. {@code '.txt'}, {@code '.jpg'}, {@code '.pdf'};
* if the filename extension cannot be determined, an empty {@link Optional} is returned
*/
@SuppressWarnings({"unchecked", "squid:S1612", "squid:S1166", "PMD.LambdaCanBeMethodReference"})
default Optional filenameExtension() {
MimeTypes defaultMimeTypes = MimeTypes.getDefaultMimeTypes();
try {
MimeType mimeType = defaultMimeTypes.forName(mimeType());
String extension = mimeType.getExtension();
boolean isValidExtension = extension.isBlank();
return Conditional.conditional(isValidExtension)
.onTrue(() -> Optional.empty())
.onFalse(() -> Optional.of(extension))
.get(Optional.class);
} catch (MimeTypeException exception) {
return Optional.empty();
}
}
/**
* Creates a new instance of {@link AssetMetadata} that has a property of the
* associated {@link Asset} specified by the passed parameters set.
* @param key name of a property of the associated {@link Asset}
* @param value value of a new property of the associated {@link Asset}
* @return new instance of {@link AssetMetadata} that has a property of the
* associated {@link Asset} specified by the passed parameters set
*/
default AssetMetadata set(String key, String value) {
Map newValues = new ConcurrentHashMap<>(all());
newValues.put(key, value);
String mimeType = mimeType();
Optional properties = properties().flatMap(
nodeProperties -> nodeProperties.setProperty(key, value)
);
return new AssetMetadata() {
@Override
public String mimeType() {
return mimeType;
}
@Override
public Map all() {
return Collections.unmodifiableMap(newValues);
}
@Override
public Optional properties() {
return properties;
}
};
}
/**
* If this {@link AssetMetadata} is backed by a {@link Node} of type {@link Asset#NT_ASSET_METADATA},
* returns an {@link Optional} containing {@link NodeProperties} of that {@link Node};
* otherwise, an empty {@link Optional} is returned.
* @return if this {@link AssetMetadata} is backed by a {@link Node} of type {@link Asset#NT_ASSET_METADATA},
* returns an {@link Optional} containing {@link NodeProperties} of that {@link Node};
* otherwise, an empty {@link Optional} is returned
*/
Optional properties();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy