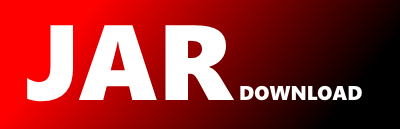
eu.ciechanowiec.sling.rocket.asset.FileMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sling.rocket.commons Show documentation
Show all versions of sling.rocket.commons Show documentation
Common utilities used by Sling Rocket
package eu.ciechanowiec.sling.rocket.asset;
import eu.ciechanowiec.sling.rocket.jcr.NodeProperties;
import eu.ciechanowiec.sneakyfun.SneakySupplier;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
import org.apache.tika.Tika;
import java.io.File;
import java.util.Map;
import java.util.Optional;
import java.util.function.Supplier;
/**
* In-memory {@link AssetMetadata} generated for a {@link File}. The mime type is detected automatically.
*/
@Slf4j
@ToString
public class FileMetadata implements AssetMetadata {
@ToString.Exclude
private final Supplier mimeTypeSupplier;
@ToString.Exclude
private final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy