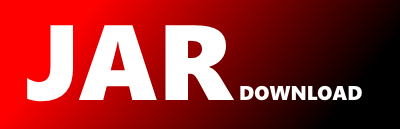
eu.dicodeproject.analysis.hbase.TweetCols Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integration Show documentation
Show all versions of integration Show documentation
The examples module provides glue code implementation for extracting common phrases, key word distributions and more from tweets stored on HDFS/HBase. It builds on Mahout for more sophisticated analysis.
The newest version!
/**
* Copyright (C) 2010, 2011 Neofonie GmbH
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.dicodeproject.analysis.hbase;
import org.apache.hadoop.hbase.util.Bytes;
public enum TweetCols {
/** Column names. */
CREATION_DATE { private byte[] val = Bytes.toBytes("creationDate"); public byte[] bytes() { return val; } },
FROM { private byte[] val = Bytes.toBytes("from"); public byte[] bytes() { return val; } },
FROM_ID { private byte[] val = Bytes.toBytes("fromId"); public byte[] bytes() { return val; } },
GEO { private byte[] val = Bytes.toBytes("geo"); public byte[] bytes() { return val; } },
IMAGE_URL { private byte[] val = Bytes.toBytes("imageUrl"); public byte[] bytes() { return val; } },
LANG { private byte[] val = Bytes.toBytes("lang"); public byte[] bytes() { return val; } },
SOURCE { private byte[] val = Bytes.toBytes("source"); public byte[] bytes() { return val; } },
TEXT { private byte[] val = Bytes.toBytes("text"); public byte[] bytes() { return val; } },
TO { private byte[] val = Bytes.toBytes("to"); public byte[] bytes() { return val; } },
HASHTAGS { private byte[] val = Bytes.toBytes("hashtags"); public byte[] bytes() { return val; } },
IN_REPLY_TO_STATUS_ID { private byte[] val = Bytes.toBytes("inReplyToStatusId"); public byte[] bytes() { return val; } },
PLACE_COUNTRY_CODE { private byte[] val = Bytes.toBytes("placeCountryCode"); public byte[] bytes() { return val; } },
PLACE_FULL_NAME { private byte[] val = Bytes.toBytes("placeFullName"); public byte[] bytes() { return val; } },
PLACE_TYPE { private byte[] val = Bytes.toBytes("placeType"); public byte[] bytes() { return val; } },
PLACE_LATITUDE { private byte[] val = Bytes.toBytes("placeLatitude"); public byte[] bytes() { return val; } },
PLACE_LONGITUDE { private byte[] val = Bytes.toBytes("placeLongitude"); public byte[] bytes() { return val; } },
RETWEET_COUNT { private byte[] val = Bytes.toBytes("retweetCount"); public byte[] bytes() { return val; } },
URL { private byte[] val = Bytes.toBytes("url"); public byte[] bytes() { return val; } },
USER_IDS_MENTIONED { private byte[] val = Bytes.toBytes("userIdsMentioned"); public byte[] bytes() { return val; } },
USER_NAMES_MENTIONED { private byte[] val = Bytes.toBytes("userNamesMentioned"); public byte[] bytes() { return val; } },
// user table
USER_CREATED_AT { private byte[] val = Bytes.toBytes("userCreatedAt"); public byte[] bytes() { return val; } },
USER_DESCRIPTION { private byte[] val = Bytes.toBytes("userDescription"); public byte[] bytes() { return val; } },
USER_FAVOURITES_COUNT { private byte[] val = Bytes.toBytes("userFavouritesCount"); public byte[] bytes() { return val; } },
USER_FOLLOWERS_COUNT { private byte[] val = Bytes.toBytes("userFollowersCount"); public byte[] bytes() { return val; } },
USER_FRIENDS_COUNT { private byte[] val = Bytes.toBytes("userFriendsCount"); public byte[] bytes() { return val; } },
USER_LISTED_COUNT { private byte[] val = Bytes.toBytes("userListedCount"); public byte[] bytes() { return val; } },
USER_LOCATION { private byte[] val = Bytes.toBytes("userLocation"); public byte[] bytes() { return val; } },
USER_NAME { private byte[] val = Bytes.toBytes("userName"); public byte[] bytes() { return val; } },
USER_PROFILE_BACKGROUND_COLOR { private byte[] val = Bytes.toBytes("userProfileBackgroundColor"); public byte[] bytes() { return val; } },
USER_PROFILE_BACKGROUND_IMAGE_URL { private byte[] val = Bytes.toBytes("userProfileBackgroundImageUrl"); public byte[] bytes() { return val; } },
USER_PROFILE_IMAGE_URL { private byte[] val = Bytes.toBytes("userProfileImageURL"); public byte[] bytes() { return val; } },
USER_PROFILE_LINK_COLOR { private byte[] val = Bytes.toBytes("userProfileLinkColor"); public byte[] bytes() { return val; } },
USER_PROFILE_SIDEBAR_BORDER_COLOR { private byte[] val = Bytes.toBytes("userProfileSidebarBorderColor"); public byte[] bytes() { return val; } },
USER_PROFILE_SIDEBAR_FILL_COLOR { private byte[] val = Bytes.toBytes("userProfileSidebarFillColor"); public byte[] bytes() { return val; } },
USER_PROFILE_TEXT_COLOR { private byte[] val = Bytes.toBytes("userProfileTextColor"); public byte[] bytes() { return val; } },
USER_SCREEN_NAME { private byte[] val = Bytes.toBytes("userScreenName"); public byte[] bytes() { return val; } },
USER_STATUSES_COUNT { private byte[] val = Bytes.toBytes("userStatusesCount"); public byte[] bytes() { return val; } },
USER_TIMEZONE { private byte[] val = Bytes.toBytes("userTimezone"); public byte[] bytes() { return val; } },
USER_URL { private byte[] val = Bytes.toBytes("userURL"); public byte[] bytes() { return val; } },
USER_UTC_OFFSET { private byte[] val = Bytes.toBytes("userUtcOffset"); public byte[] bytes() { return val; } };
public abstract byte[] bytes ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy