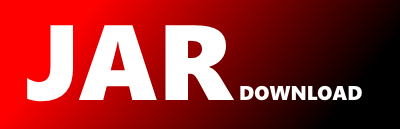
eu.dicodeproject.analysis.lucene.TweetAnalyzer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integration Show documentation
Show all versions of integration Show documentation
The examples module provides glue code implementation for extracting common phrases, key word distributions and more from tweets stored on HDFS/HBase. It builds on Mahout for more sophisticated analysis.
The newest version!
/**
* Copyright (C) 2010, 2011 Neofonie GmbH
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.dicodeproject.analysis.lucene;
import eu.dicodeproject.analysis.util.Language;
import java.io.Reader;
import java.util.Set;
import org.apache.lucene.analysis.LowerCaseFilter;
import org.apache.lucene.analysis.ReusableAnalyzerBase;
import org.apache.lucene.analysis.StopFilter;
import org.apache.lucene.analysis.StopwordAnalyzerBase;
import org.apache.lucene.analysis.TokenStream;
import org.apache.lucene.analysis.Tokenizer;
import org.apache.lucene.analysis.en.EnglishPossessiveFilter;
import org.apache.lucene.analysis.fr.ElisionFilter;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.analysis.standard.StandardFilter;
import org.apache.lucene.analysis.standard.UAX29URLEmailTokenizer;
import org.apache.lucene.analysis.tr.TurkishLowerCaseFilter;
import org.apache.lucene.util.Version;
/**
* @author jakob
*/
public class TweetAnalyzer extends StopwordAnalyzerBase {
private Language language;
public TweetAnalyzer(Language language, Version matchVersion, Set> stopwords) {
super(matchVersion, stopwords);
this.language = language;
}
public TweetAnalyzer(Language language, Version matchVersion) {
this(language, matchVersion, StandardAnalyzer.STOP_WORDS_SET);
}
public TweetAnalyzer(String langCode, Version matchVersion, Set> stopwords) {
this(Language.fromCode(langCode), matchVersion, stopwords);
}
public TweetAnalyzer(String langCode, Version matchVersion) {
this(Language.fromCode(langCode), matchVersion, StandardAnalyzer.STOP_WORDS_SET);
}
@Override
public TokenStreamComponents createComponents(String fieldName, Reader reader) {
// tokenizer
Tokenizer source = new UAX29URLEmailTokenizer(reader);
// standard filter
TokenStream result = new StandardFilter(matchVersion, source);
// langCode specific filters
if (matchVersion.onOrAfter(Version.LUCENE_31)) {
if (language == Language.ENGLISH) {
result = new EnglishPossessiveFilter(result);
}
else if (language == Language.FRENCH) {
result = new ElisionFilter(matchVersion, result);
}
}
// lower-casing
if (language == Language.TURKISH) {
result = new TurkishLowerCaseFilter(result);
}
else {
result = new LowerCaseFilter(matchVersion, result);
}
// stop-wording
result = new StopFilter(matchVersion, result, stopwords);
// // stemming exclusions
// if(!stemExclusionSet.isEmpty()) {
// result = new KeywordMarkerFilter(result, stemExclusionSet);
// }
// stemming
result = StemmerFactory.getStemmer(language, matchVersion, result);
// done
return new ReusableAnalyzerBase.TokenStreamComponents(source, result);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy