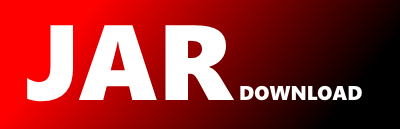
eu.dicodeproject.analysis.twitter.SchemaUpdaterDriver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integration Show documentation
Show all versions of integration Show documentation
The examples module provides glue code implementation for extracting common phrases, key word distributions and more from tweets stored on HDFS/HBase. It builds on Mahout for more sophisticated analysis.
The newest version!
/**
* Copyright (C) 2010, 2011 Neofonie GmbH
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
*
*/
package eu.dicodeproject.analysis.twitter;
import java.io.IOException;
import java.util.Map;
import org.apache.commons.lang.StringUtils;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.output.NullOutputFormat;
import org.apache.hadoop.util.ToolRunner;
import org.apache.mahout.common.AbstractJob;
/**
*
*/
public class SchemaUpdaterDriver extends AbstractJob {
public static void main(String args[]) throws Exception {
ToolRunner.run(new SchemaUpdaterDriver(), args);
}
@Override
public int run(String[] args) throws ClassNotFoundException, InterruptedException, IOException {
// source
addOption("sourcetable", "s", "The hbase table holding our data.", "twitterstream");
addOption("sourcetextfamily", "stf", "The source table's column family holding our data.", "textFamily");
addOption("sourcemetafamily", "smf", "The source table's column family holding our data.", "metaFamily");
// sink
addOption("table", "t", "The hbase table holding our data.");
addOption("family", "f", "The column family holding our data.", "d");
Map argMap = parseArguments(args);
if (argMap == null) {
return 1;
}
String sourceTable = argMap.get("--sourcetable");
String sourceTextFamily = argMap.get("--sourcetextfamily");
String sourceMetaFamily = argMap.get("--sourcemetafamily");
String table = argMap.get("--table");
if (StringUtils.isBlank(table)) {
return 2;
}
String family = argMap.get("--family");
Configuration cfg = HBaseConfiguration.create();
cfg.set("sourceTextFamily", sourceTextFamily);
cfg.set("sourceMetaFamily", sourceMetaFamily);
cfg.set("table", table);
cfg.set("family", family);
Job job = new Job(cfg);
job.setJarByClass(SchemaUpdaterDriver.class);
Scan scan = new Scan();
TableMapReduceUtil.initTableMapperJob(sourceTable, scan, SchemaUpdaterMapper.class, NullWritable.class,
NullWritable.class, job);
job.setJobName("SchemaUpdater");
job.setMapOutputKeyClass(NullWritable.class);
job.setMapOutputValueClass(NullWritable.class);
// identity reducer
job.setReducerClass(Reducer.class);
job.setOutputKeyClass(NullWritable.class);
job.setOutputValueClass(NullWritable.class);
job.setOutputFormatClass(NullOutputFormat.class);
job.setNumReduceTasks(0);
job.waitForCompletion(true);
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy