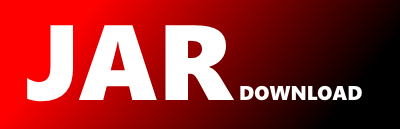
jdplus.sa.base.csv.CsvInformationFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdplus-sa-base-csv Show documentation
Show all versions of jdplus-sa-base-csv Show documentation
${project.parent.artifactId} - ${project.artifactId}
/*
* Copyright 2013 National Bank of Belgium
*
* Licensed under the EUPL, Version 1.1 or – as soon they will be approved
* by the European Commission - subsequent versions of the EUPL (the "Licence");
* You may not use this work except in compliance with the Licence.
* You may obtain a copy of the Licence at:
*
* http://ec.europa.eu/idabc/eupl
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the Licence is distributed on an "AS IS" basis,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the Licence for the specific language governing permissions and
* limitations under the Licence.
*/
package jdplus.sa.base.csv;
import jdplus.toolkit.base.api.arima.SarimaOrders;
import jdplus.toolkit.base.api.data.Parameter;
import jdplus.toolkit.base.api.information.Explorable;
import jdplus.toolkit.base.api.information.Information;
import jdplus.toolkit.base.api.information.InformationSet;
import jdplus.toolkit.base.api.information.formatters.*;
import jdplus.toolkit.base.api.math.Complex;
import jdplus.toolkit.base.api.processing.ProcDiagnostic;
import jdplus.toolkit.base.api.stats.StatisticalTest;
import jdplus.toolkit.base.api.timeseries.TsPeriod;
import jdplus.toolkit.base.api.timeseries.regression.RegressionItem;
import jdplus.toolkit.base.api.util.MultiLineNameUtil;
import jdplus.toolkit.base.api.util.NamedObject;
import jdplus.toolkit.base.api.util.WildCards;
import nbbrd.picocsv.Csv;
import java.io.IOException;
import java.io.Writer;
import java.lang.reflect.Type;
import java.text.DecimalFormat;
import java.util.*;
import java.util.Map.Entry;
/**
*
*/
public final class CsvInformationFormatter {
private static final HashMap DICTIONARY = new HashMap<>();
private static final String NEWLINE = System.lineSeparator();
private static volatile Character CSV_SEPARATOR;
private static Locale LOCALE;
static {
LOCALE = Locale.getDefault();
DecimalFormat fmt = (DecimalFormat) DecimalFormat.getNumberInstance(LOCALE);
fmt.setMaximumFractionDigits(BasicConfiguration.getFractionDigits());
fmt.setGroupingUsed(false);
char sep = fmt.getDecimalFormatSymbols().getDecimalSeparator();
if (sep == ',') {
CSV_SEPARATOR = ';';
} else {
CSV_SEPARATOR = ',';
}
DICTIONARY.put(double.class, new DoubleFormatter());
DICTIONARY.put(int.class, new IntegerFormatter());
DICTIONARY.put(long.class, new LongFormatter());
DICTIONARY.put(boolean.class, new BooleanFormatter("1", "0"));
DICTIONARY.put(Double.class, new DoubleFormatter());
DICTIONARY.put(Integer.class, new IntegerFormatter());
DICTIONARY.put(Long.class, new LongFormatter());
DICTIONARY.put(Boolean.class, new BooleanFormatter("1", "0"));
DICTIONARY.put(Complex.class, new ComplexFormatter());
DICTIONARY.put(String.class, new StringFormatter());
DICTIONARY.put(String[].class, new StringArrayFormatter());
DICTIONARY.put(SarimaOrders.class, new SarimaFormatter());
DICTIONARY.put(Parameter.class, new ParameterFormatter());
DICTIONARY.put(TsPeriod.class, new PeriodFormatter());
DICTIONARY.put(RegressionItem.class, new RegressionItemFormatter(true));
DICTIONARY.put(StatisticalTest.class, new StatisticalTestFormatter());
DICTIONARY.put(ProcDiagnostic.class, new DiagnosticFormatter());
}
private CsvInformationFormatter() {
throw new UnsupportedOperationException("This is a utility class and cannot be instantiated");
}
public static char getCsvSeparator() {
return CSV_SEPARATOR;
}
public static void setCsvSeparator(Character c) {
CSV_SEPARATOR = c;
}
public static Locale getLocale() {
return LOCALE;
}
public static void setLocale(Locale locale) {
CsvInformationFormatter.LOCALE = locale;
}
public static Set formattedTypes() {
return DICTIONARY.keySet();
}
// preparing the matrix:
// for each record, for each name, we search for the length of an item, the actual items (in case of
// wildcards) and the corresponding result
private static class MatrixItem {
static MatrixItem ofInformationSet(String id, InformationSet record, boolean shortname) {
MatrixItem result = new MatrixItem();
result.fill(id, record, shortname);
return result;
}
static MatrixItem ofExplorable(String id, Explorable record, boolean shortname) {
MatrixItem result = new MatrixItem();
result.fill(id, record, shortname);
return result;
}
private static final Object[] EMPTY = new Object[0];
private static final String[] SEMPTY = new String[0];
int length;
String[] items;
Object[] results = EMPTY;
boolean isHomogeneous() {
if (results.length <= 1) {
return true;
}
Class c = null;
for (int i = 0; i < results.length; ++i) {
if (results[i] != null) {
if (c == null) {
c = results[i].getClass();
} else if (!results[i].getClass().equals(c)) {
return false;
}
}
}
return true;
}
void fill(final String id, InformationSet record, boolean shortname) {
int l = id.indexOf(':');
String sid = id;
if (l >= 0) {
sid = id.substring(0, l);
String s1 = id.substring(l + 1);
try {
length = Integer.parseInt(s1);
} catch (Exception ex) {
length = 1;
}
}
if (WildCards.hasWildCards(sid)) {
List> sel = record.select(sid);
if (!sel.isEmpty()) {
int n = sel.size();
results = new Object[n];
items = new String[n];
for (int i = 0; i < n; ++i) {
Information
© 2015 - 2025 Weber Informatics LLC | Privacy Policy