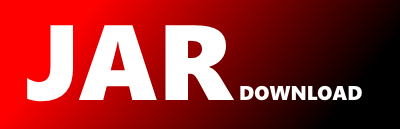
internal.sql.base.api.SqlTableAsCubeUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdplus-sql-base-api Show documentation
Show all versions of jdplus-sql-base-api Show documentation
${project.parent.artifactId} - ${project.artifactId}
/*
* Copyright 2017 National Bank of Belgium
*
* Licensed under the EUPL, Version 1.1 or - as soon they will be approved
* by the European Commission - subsequent versions of the EUPL (the "Licence");
* You may not use this work except in compliance with the Licence.
* You may obtain a copy of the Licence at:
*
* http://ec.europa.eu/idabc/eupl
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the Licence is distributed on an "AS IS" basis,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the Licence for the specific language governing permissions and
* limitations under the Licence.
*/
package internal.sql.base.api;
import jdplus.toolkit.base.tsp.cube.TableAsCubeConnection.AllSeriesCursor;
import jdplus.toolkit.base.tsp.cube.TableAsCubeConnection.AllSeriesWithDataCursor;
import jdplus.toolkit.base.tsp.cube.TableAsCubeConnection.ChildrenCursor;
import jdplus.toolkit.base.tsp.cube.TableAsCubeConnection.SeriesCursor;
import jdplus.toolkit.base.tsp.cube.TableAsCubeConnection.SeriesWithDataCursor;
import java.sql.ResultSet;
import java.util.Date;
/**
*
* @author Philippe Charles
*/
@lombok.experimental.UtilityClass
public class SqlTableAsCubeUtil {
public AllSeriesCursor allSeriesCursor(ResultSet rs, AutoCloseable closeable, ResultSetFunc toDimValues, ResultSetFunc toLabel) {
return new ResultSetAllSeriesCursor(rs, closeable, toDimValues, toLabel);
}
public AllSeriesWithDataCursor allSeriesWithDataCursor(ResultSet rs, AutoCloseable closeable, ResultSetFunc toDimValues, ResultSetFunc toPeriod, ResultSetFunc toValue, ResultSetFunc toLabel) {
return new ResultSetAllSeriesWithDataCursor(rs, closeable, toDimValues, toPeriod, toValue, toLabel);
}
public SeriesCursor seriesCursor(ResultSet rs, AutoCloseable closeable, ResultSetFunc toLabel) {
return new ResultSetSeriesCursor(rs, closeable, toLabel);
}
public SeriesWithDataCursor seriesWithDataCursor(ResultSet rs, AutoCloseable closeable, ResultSetFunc toPeriod, ResultSetFunc toValue, ResultSetFunc toLabel) {
return new ResultSetSeriesWithDataCursor(rs, closeable, toPeriod, toValue, toLabel);
}
public ChildrenCursor childrenCursor(ResultSet rs, AutoCloseable closeable, ResultSetFunc toChild) {
return new ResultSetChildrenCursor(rs, closeable, toChild);
}
//
@lombok.RequiredArgsConstructor
private static final class ResultSetAllSeriesCursor implements AllSeriesCursor {
private final ResultSet rs;
@lombok.experimental.Delegate
private final AutoCloseable closeable;
private final ResultSetFunc toDimValues;
private final ResultSetFunc toLabel;
@lombok.Getter
private String[] dimValues = null;
@lombok.Getter
private String labelOrNull = null;
@Override
public boolean nextRow() throws Exception {
if (rs.next()) {
dimValues = toDimValues.applyWithSql(rs);
labelOrNull = toLabel.applyWithSql(rs);
return true;
}
return false;
}
}
@lombok.RequiredArgsConstructor
private static final class ResultSetAllSeriesWithDataCursor implements AllSeriesWithDataCursor {
private final ResultSet rs;
@lombok.experimental.Delegate
private final AutoCloseable closeable;
private final ResultSetFunc toDimValues;
private final ResultSetFunc toPeriod;
private final ResultSetFunc toValue;
private final ResultSetFunc toLabel;
@lombok.Getter
private String[] dimValues = null;
@lombok.Getter
private java.util.Date periodOrNull = null;
@lombok.Getter
private Number valueOrNull = null;
@lombok.Getter
private String labelOrNull = null;
@Override
public boolean nextRow() throws Exception {
if (rs.next()) {
dimValues = toDimValues.applyWithSql(rs);
periodOrNull = toPeriod.applyWithSql(rs);
valueOrNull = periodOrNull != null ? toValue.applyWithSql(rs) : null;
labelOrNull = toLabel.applyWithSql(rs);
return true;
}
return false;
}
}
@lombok.RequiredArgsConstructor
private static final class ResultSetSeriesCursor implements SeriesCursor {
private final ResultSet rs;
@lombok.experimental.Delegate
private final AutoCloseable closeable;
private final ResultSetFunc toLabel;
@lombok.Getter
private String labelOrNull = null;
@Override
public boolean nextRow() throws Exception {
if (rs.next()) {
labelOrNull = toLabel.applyWithSql(rs);
return true;
}
return false;
}
}
@lombok.RequiredArgsConstructor
private static final class ResultSetSeriesWithDataCursor implements SeriesWithDataCursor {
private final ResultSet rs;
@lombok.experimental.Delegate
private final AutoCloseable closeable;
private final ResultSetFunc toPeriod;
private final ResultSetFunc toValue;
private final ResultSetFunc toLabel;
@lombok.Getter
private java.util.Date periodOrNull = null;
@lombok.Getter
private Number valueOrNull = null;
@lombok.Getter
private String labelOrNull = null;
@Override
public boolean nextRow() throws Exception {
if (rs.next()) {
periodOrNull = toPeriod.applyWithSql(rs);
valueOrNull = periodOrNull != null ? toValue.applyWithSql(rs) : null;
labelOrNull = toLabel.applyWithSql(rs);
return true;
}
return false;
}
}
@lombok.RequiredArgsConstructor
private static final class ResultSetChildrenCursor implements ChildrenCursor {
private final ResultSet rs;
@lombok.experimental.Delegate
private final AutoCloseable closeable;
private final ResultSetFunc toChild;
@lombok.Getter
private String child = null;
@Override
public boolean nextRow() throws Exception {
if (rs.next()) {
child = toChild.applyWithSql(rs);
if (child == null) {
child = NULL_VALUE;
}
return true;
}
return false;
}
private static final String NULL_VALUE = "";
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy