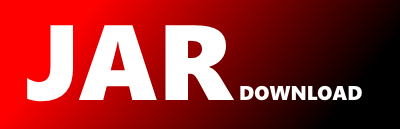
jdplus.x13.base.xml.XmlOutliersSpec Maven / Gradle / Ivy
Show all versions of jdplus-x13-base-xml Show documentation
/*
* Copyright 2016 National Bank of Belgium
*
* Licensed under the EUPL, Version 1.1 or – as soon they will be approved
* by the European Commission - subsequent versions of the EUPL (the "Licence");
* You may not use this work except in compliance with the Licence.
* You may obtain a copy of the Licence at:
*
* http://ec.europa.eu/idabc/eupl
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the Licence is distributed on an "AS IS" basis,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the Licence for the specific language governing permissions and
* limitations under the Licence.
*/
package jdplus.x13.base.xml;
import jdplus.toolkit.base.xml.legacy.modelling.XmlOutlierSpec;
import jdplus.x13.base.api.regarima.OutlierSpec;
import jdplus.x13.base.api.regarima.SingleOutlierSpec;
import jdplus.toolkit.base.xml.legacy.core.XmlPeriodSelection;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlList;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
*
* Java class for OutlierSpecType complex type.
*
*
* The following schema fragment specifies the expected content contained within
* this class.
*
*
* <complexType name="OutlierSpecType">
* <complexContent>
* <extension base="{ec/eurostat/jdemetra/modelling}OutlierSpecType">
* <sequence>
* <element name="Types" type="{http://www.w3.org/2001/XMLSchema}NMTOKENS"/>
* <element name="CriticalValue" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}double">
* <minInclusive value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="EML" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="DeltaTC" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}double">
* <minExclusive value="0"/>
* <maxExclusive value="1"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "OutlierSpecType", propOrder = {
"types",
"criticalValue",
"outlier",
"deltaTC",
"method"
})
public class XmlOutliersSpec
extends XmlOutlierSpec {
@XmlList
@XmlElement(name = "Types", required = true)
@XmlSchemaType(name = "NMTOKENS")
protected List types;
@XmlElement(name = "CriticalValue")
protected Double criticalValue;
@XmlElement(name = "Outlier")
protected List outlier;
@XmlElement(name = "DeltaTC", defaultValue = "0.7")
protected Double deltaTC;
@XmlElement(name = "Method")
protected OutlierSpec.Method method;
/**
* Gets the value of the types property.
*
*
* This accessor method returns a reference to the live list, not a
* snapshot. Therefore any modification you make to the returned list will
* be present inside the JAXB object. This is why there is not a
* set
method for the types property.
*
*
* For example, to add a new item, do as follows:
*
* getTypes().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list {@link String }
*
*
*/
public List getTypes() {
if (types == null) {
types = new ArrayList<>();
}
return this.types;
}
/**
* Gets the value of the criticalValue property.
*
* @return possible object is {@link Double }
*
*/
public Double getCriticalValue() {
return criticalValue;
}
/**
* Sets the value of the criticalValue property.
*
* @param value allowed object is {@link Double }
*
*/
public void setCriticalValue(Double value) {
if (value != null && value == 0) {
this.criticalValue = null;
} else {
this.criticalValue = value;
}
}
/**
* Gets the value of the deltaTC property.
*
* @return possible object is {@link Double }
*
*/
public double getDeltaTC() {
return deltaTC == null ? OutlierSpec.DEF_TCRATE : deltaTC;
}
/**
* Sets the value of the deltaTC property.
*
* @param value allowed object is {@link Double }
*
*/
public void setDeltaTC(Double value) {
if (value != null && value == OutlierSpec.DEF_TCRATE) {
deltaTC = null;
} else {
this.deltaTC = value;
}
}
public List getOutliers() {
if (outlier == null) {
outlier = new ArrayList<>();
}
return outlier;
}
public OutlierSpec.Method getMethod() {
return method;
}
public void setMethod(OutlierSpec.Method method) {
if (method != null && method == OutlierSpec.Method.AddOne) {
this.method = null;
} else {
this.method = method;
}
}
public static final OutlierSpec unmarshal(XmlOutliersSpec xml) {
if (xml == null) {
return OutlierSpec.DEFAULT_DISABLED;
}
OutlierSpec.Builder builder = OutlierSpec.builder();
if (xml.span != null) {
builder = builder.span(XmlPeriodSelection.unmarshal(xml.span));
}
if (xml.types != null && !xml.types.isEmpty()) {
double cv = xml.criticalValue != null ? xml.criticalValue : 0;
for (String o : xml.types) {
builder.type(new SingleOutlierSpec(o, cv));
}
} else if (xml.outlier != null) {
// process single outliers
for (XmlSingleOutlierSpec xcur : xml.outlier) {
SingleOutlierSpec cur = new SingleOutlierSpec(xcur.type,
xcur.criticalValue != null ? xcur.criticalValue : 0);
builder = builder.type(cur);
}
}
if (xml.deltaTC != null) {
builder.monthlyTCRate(xml.deltaTC);
}
if (xml.method != null) {
builder.method(xml.method);
}
return builder.build();
}
public static XmlOutliersSpec marshal(OutlierSpec v) {
if (!v.isUsed()) {
return null;
}
XmlOutliersSpec xml = new XmlOutliersSpec();
marshal(v, xml);
return xml;
}
public static boolean marshal(OutlierSpec v, XmlOutliersSpec xml) {
xml.span = XmlPeriodSelection.marshal(v.getSpan());
xml.setCriticalValue(v.getDefaultCriticalValue());
xml.setDeltaTC(v.getMonthlyTCRate());
List otypes = v.getTypes();
double va=otypes .get(0).getCriticalValue();
boolean same = true;
for (int i = 1; i < otypes.size(); ++i) {
if (otypes.get(i).getCriticalValue() != va) {
same = false;
break;
}
}
if (same) {
List stypes = xml.getTypes();
for (int i = 0; i < otypes.size(); ++i) {
stypes.add(otypes.get(i).getType());
}
} else {
List xtypes = xml.getOutliers();
for (SingleOutlierSpec o : otypes) {
XmlSingleOutlierSpec xcur = XmlSingleOutlierSpec.marshal(o);
xtypes.add(xcur);
}
}
return true;
}
;
}