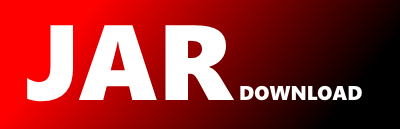
eu.europa.esig.dss.model.ManifestFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dss-model Show documentation
Show all versions of dss-model Show documentation
DSS Model contains the data model representation for DSS
/**
* DSS - Digital Signature Services
* Copyright (C) 2015 European Commission, provided under the CEF programme
*
* This file is part of the "DSS - Digital Signature Services" project.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
package eu.europa.esig.dss.model;
import eu.europa.esig.dss.enumerations.ASiCManifestTypeEnum;
import eu.europa.esig.dss.enumerations.DigestAlgorithm;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* Represents a parsed Manifest File object
*/
public class ManifestFile implements Serializable {
private static final long serialVersionUID = -5971045309587760817L;
private static final Logger LOG = LoggerFactory.getLogger(ManifestFile.class);
/** The DSSDocument represented by the ManifestFile */
private DSSDocument document;
/** The name of a signature or timestamp associated to the ManifestFile */
private String signatureFilename;
/** List of entries present in the document */
private List entries;
/** Defines the type of the manifest document */
private ASiCManifestTypeEnum manifestType;
/**
* Default constructor instantiating object with null values
*/
public ManifestFile() {
// empty
}
/**
* Gets the {@code DSSDocument} representing the manifest
*
* @return {@link DSSDocument}
*/
public DSSDocument getDocument() {
return document;
}
/**
* Sets the manifest document
*
* @param document {@link DSSDocument}
*/
public void setDocument(DSSDocument document) {
this.document = document;
}
/**
* Gets the manifest document's filename
*
* @return {@link String}
*/
public String getFilename() {
return document.getName();
}
/**
* Gets the signature filename
*
* @return {@link String}
*/
public String getSignatureFilename() {
return signatureFilename;
}
/**
* Sets the signature filename
*
* @param signatureFilename {@link String}
*/
public void setSignatureFilename(String signatureFilename) {
this.signatureFilename = signatureFilename;
}
/**
* Gets base64 encoded digest string of the manifest document for the given {@code digestAlgorithm}
*
* @param digestAlgorithm {@link DigestAlgorithm} to compute digest
* @return {@link String} base64-encoded digest value
*/
public String getDigestBase64String(DigestAlgorithm digestAlgorithm) {
return document.getDigest(digestAlgorithm);
}
/**
* Gets a list of {@code ManifestEntry}s
*
* @return a list of {@code ManifestEntry}s
*/
public List getEntries() {
if (entries == null) {
entries = new ArrayList<>();
}
return entries;
}
/**
* Sets a list of {@code ManifestEntry}s
*
* @param entries a list of {@code ManifestEntry}s
*/
public void setEntries(List entries) {
this.entries = entries;
}
/**
* Gets a type of the ASiC Manifest file
*
* @return {@link ASiCManifestTypeEnum}
*/
public ASiCManifestTypeEnum getManifestType() {
return manifestType;
}
/**
* Sets a type of the ASiC Manifest file
*
* @param manifestType {@link ASiCManifestTypeEnum}
*/
public void setManifestType(ASiCManifestTypeEnum manifestType) {
this.manifestType = manifestType;
}
/**
* Gets if the manifest is related to a timestamp
*
* @return TRUE if it is a timestamp's manifest, FALSE otherwise
* @deprecated since DSS 5.13. Use {@code #getManifestType} method instead.
*/
@Deprecated
public boolean isTimestampManifest() {
return manifestType != null && ASiCManifestTypeEnum.TIMESTAMP == manifestType;
}
/**
* Sets if the manifest is related to a timestamp
*
* @param timestampManifest if it is a timestamp's manifest
* @deprecated since DSS 5.13. Use {@code #setManifestType} method instead.
*/
@Deprecated
public void setTimestampManifest(boolean timestampManifest) {
LOG.warn("Use of deprecated method #setTimestampManifest! Use #setManifestType instead.");
setManifestType(ASiCManifestTypeEnum.TIMESTAMP);
}
/**
* Gets if the manifest is related to an archive timestamp (ASiC-E with CAdES)
*
* @return TRUE if it is an archive timestamp's manifest, FALSE otherwise
* @deprecated since DSS 5.13. Use {@code #getManifestType} method instead.
*/
@Deprecated
public boolean isArchiveManifest() {
return manifestType != null && ASiCManifestTypeEnum.ARCHIVE_MANIFEST == manifestType;
}
/**
* Sets if the manifest is related to an archive timestamp (ASiC-E with CAdES)
*
* @param archiveManifest if it is an archive timestamp's manifest
* @deprecated since DSS 5.13. Use {@code #setManifestType} method instead.
*/
@Deprecated
public void setArchiveManifest(boolean archiveManifest) {
LOG.warn("Use of deprecated method #setTimestampManifest! Use #setManifestType instead.");
setManifestType(ASiCManifestTypeEnum.ARCHIVE_MANIFEST);
}
/**
* Returns a {@link ManifestEntry} with argument Rootfile="true"
*
* @return {@link ManifestEntry} if the rootfile is found, FALSE otherwise
*/
public ManifestEntry getRootFile() {
for (ManifestEntry entry : getEntries()) {
if (entry.isRootfile()) {
return entry;
}
}
return null;
}
/**
* Checks if the document with {@code documentName} is covered by the Manifest
*
* @param documentName {@link String} to check
* @return TRUE if the document with the given name is covered, FALSE otherwise
*/
public boolean isDocumentCovered(String documentName) {
if (documentName != null && documentName.length() > 0) {
for (ManifestEntry entry : getEntries()) {
if (documentName.equals(entry.getFileName())) {
return true;
}
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy