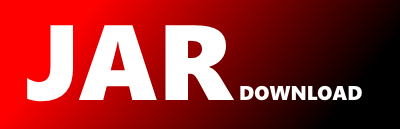
eu.fbk.twm.utils.FrequencyHashSet Maven / Gradle / Ivy
The newest version!
package eu.fbk.twm.utils;
import java.io.Serializable;
import java.util.*;
/**
* Created with IntelliJ IDEA.
* User: aprosio
* Date: 1/15/13
* Time: 5:56 PM
* To change this template use File | Settings | File Templates.
*/
public class FrequencyHashSet implements Serializable {
private HashMap support = new HashMap();
static >
SortedSet> entriesSortedByValues(Map map) {
SortedSet> sortedEntries = new TreeSet>(
new Comparator>() {
@Override
public int compare(Map.Entry e1, Map.Entry e2) {
int res = e2.getValue().compareTo(e1.getValue());
return res != 0 ? res : 1;
}
}
);
sortedEntries.addAll(map.entrySet());
return sortedEntries;
}
public SortedSet> getSorted() {
return entriesSortedByValues(support);
}
public LinkedHashMap getLinked() {
SortedSet> sorted = entriesSortedByValues(support);
LinkedHashMap temp = new LinkedHashMap();
for (Object o : sorted) {
Map.Entry entry = (Map.Entry) o;
temp.put((K) entry.getKey(), (Integer) entry.getValue());
}
return temp;
}
public void add(K o, int quantity) {
int count = support.containsKey(o) ? ((Integer) support.get(o)).intValue() + quantity : quantity;
support.put(o, count);
}
public FrequencyHashSet clone() {
FrequencyHashSet ret = new FrequencyHashSet();
for (K k : support.keySet()) {
ret.add(k, support.get(k));
}
return ret;
}
public void addAll(Collection collection) {
for (K el : collection) {
add(el);
}
}
public void addAll(FrequencyHashSet frequencyHashSet) {
for (K el : frequencyHashSet.keySet()) {
add(el, frequencyHashSet.get(el));
}
}
public void remove(K o) {
support.remove(o);
}
public void add(K o) {
add(o, 1);
}
public int size() {
return support.size();
}
public K mostFrequent() {
Iterator it = support.keySet().iterator();
Integer max = null;
K o = null;
while (it.hasNext()) {
K index = (K) it.next();
if (max == null || support.get(index) > max) {
o = index;
max = support.get(index);
}
}
return o;
}
public Set keySet() {
return support.keySet();
}
public Integer get(K o) {
return support.get(o);
}
public Integer getZero(K o) {
return support.get(o) != null ? support.get(o) : 0;
}
public Integer sum() {
int total = 0;
for (K key : support.keySet()) {
total += support.get(key);
}
return total;
}
public Set keySetWithLimit(int limit) {
HashSet ret = new HashSet();
Iterator it = support.keySet().iterator();
while (it.hasNext()) {
K key = (K) it.next();
// int value = ((Integer) support.get(key)).intValue();
int value = support.get(key);
if (value >= limit) {
ret.add(key);
// ret += key.toString() + "=" + value + "\n";
}
}
// return ret.trim();
return ret;
}
public String toString() {
return support.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy