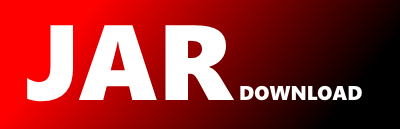
eu.fbk.twm.utils.SynchronizedCounter Maven / Gradle / Ivy
The newest version!
/*
* Copyright (2013) Fondazione Bruno Kessler (http://www.fbk.eu/)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.fbk.twm.utils;
import org.apache.log4j.Logger;
import java.io.IOException;
import java.io.Writer;
import java.util.*;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Created with IntelliJ IDEA.
* User: giuliano
* Date: 1/23/13
* Time: 11:54 AM
* To change this template use File | Settings | File Templates.
*/
public class SynchronizedCounter {
/**
* Define a static logger variable so that it references the
* Logger instance named SynchronizedCounter
.
*/
static Logger logger = Logger.getLogger(SynchronizedCounter.class.getName());
protected Map map;
protected int total;
public SynchronizedCounter() {
map = new HashMap();
}
public void addAll(Set set) {
Iterator it = set.iterator();
for (; it.hasNext(); ) {
add(it.next());
}
}
public synchronized int add(T o, int freq) {
AtomicInteger i = map.get(o);
if (i == null) {
i = new AtomicInteger(0);
map.put(o, i);
}
total += freq;
return i.addAndGet(freq);
}
public synchronized int add(T o) {
AtomicInteger i = map.get(o);
if (i == null) {
i = new AtomicInteger(0);
map.put(o, i);
}
total++;
return i.incrementAndGet();
}
public synchronized int get(T o) {
AtomicInteger i = map.get(o);
if (i == null) {
return 0;
}
return map.get(o).intValue();
}
public int total() {
return total;
}
public int size() {
return map.size();
}
public synchronized SortedMap> getSortedMap() {
SortedMap> sortedMap = new TreeMap>(new Comparator() {
public int compare(AtomicInteger e1, AtomicInteger e2) {
if (e1.intValue() > e2.intValue()) {
return -1;
}
else if (e1.intValue() < e2.intValue()) {
return 1;
}
return 0;
}
});
Iterator it = map.keySet().iterator();
while (it.hasNext()) {
T o = it.next();
AtomicInteger i = map.get(o);
List list = sortedMap.get(i);
if (list == null) {
list = new ArrayList();
list.add(o);
sortedMap.put(i, list);
}
else {
list.add(o);
}
}
return sortedMap;
}
public synchronized void write(Writer w) throws IOException {
SortedMap> sortedMap = getSortedMap();
Iterator it = sortedMap.keySet().iterator();
AtomicInteger i;
for (; it.hasNext(); ) {
i = it.next();
List list = sortedMap.get(i);
for (int j = 0; j < list.size(); j++) {
w.write(i.toString());
w.write(CharacterTable.HORIZONTAL_TABULATION);
w.write(list.get(j).toString());
w.write(CharacterTable.LINE_FEED);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy