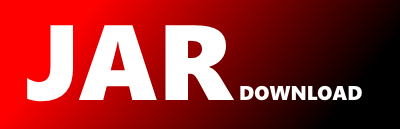
eu.fbk.twm.utils.TopicOntology Maven / Gradle / Ivy
The newest version!
package eu.fbk.twm.utils;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.OptionBuilder;
import org.apache.log4j.Logger;
import org.apache.log4j.PropertyConfigurator;
import java.io.*;
import java.util.HashMap;
/**
* Created with IntelliJ IDEA.
* User: alessio
* Date: 06/03/14
* Time: 10:55
* To change this template use File | Settings | File Templates.
*/
public class TopicOntology {
static Logger logger = Logger.getLogger(TopicOntology.class.getName());
private HashMap nodes;
public class TopicOntologyNode {
private String key, label;
public TopicOntologyNode(String key, String label) {
this.key = key;
this.label = label;
}
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
@Override
public String toString() {
return "TopicOntologyNode{" +
"key='" + key + '\'' +
", label='" + label + '\'' +
'}';
}
}
public TopicOntology() {
nodes = new HashMap<>();
}
public TopicOntology(String ontologyFile) throws IOException {
this();
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(ontologyFile), "UTF-8"));
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.startsWith("#")) {
continue;
}
if (line.length() == 0) {
continue;
}
String key = line.substring(0, 3);
String label = line.substring(3).trim();
nodes.put(key, new TopicOntologyNode(key, label));
}
reader.close();
}
@Override
public String toString() {
return "TopicOntology{" +
"nodes=" + nodes +
'}';
}
public HashMap getNodes() {
return nodes;
}
public TopicOntologyNode getNode(String key) {
return nodes.get(key);
}
public static void convertWeightedSet(WeightedSet weightedSet, TopicOntology ontology) {
if (ontology == null) {
return;
}
WeightedSet ret = new WeightedSet();
for (String key : weightedSet) {
try {
ret.add(ontology.getNode(key).getLabel(), weightedSet.get(key));
} catch (Exception ignored) {
// logger.error(key + " - " + e.getMessage());
}
}
weightedSet.clear();
for (String key : ret) {
weightedSet.add(key, ret.get(key));
}
}
public static void main(String[] args) {
CommandLineWithLogger commandLineWithLogger = new CommandLineWithLogger();
commandLineWithLogger.addOption(OptionBuilder.withDescription("Ontology file").isRequired().hasArg().withArgName("file").withLongOpt("ontology").create("o"));
CommandLine commandLine = null;
try {
commandLine = commandLineWithLogger.getCommandLine(args);
PropertyConfigurator.configure(commandLineWithLogger.getLoggerProps());
} catch (Exception e) {
System.exit(1);
}
try {
TopicOntology o = new TopicOntology(commandLine.getOptionValue("ontology"));
System.out.println(o);
} catch (Exception e) {
logger.error(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy