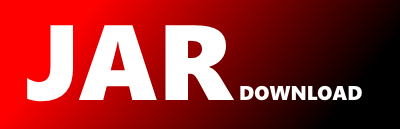
eu.future.earth.gwt.client.date.BaseDateRenderer Maven / Gradle / Ivy
package eu.future.earth.gwt.client.date;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import com.google.gwt.core.client.GWT;
import com.google.gwt.i18n.client.DateTimeFormat;
public abstract class BaseDateRenderer implements DateRenderer {
protected DateTexts dateTexts = (DateTexts) GWT.create(DateTexts.class);
public boolean gotoDayViewOnClick(){
return true;
}
public boolean headerClickable(){
return false;
}
public int getWholeEventRowHeigt(){
return 30;
}
public String getDay(Date theDate) {
DateTimeFormat formatter = DateTimeFormat.getFormat("MM/dd/yyyy");
return formatter.format(theDate);
}
public String getTime(Date theDate) {
DateTimeFormat formatter = DateTimeFormat.getFormat("HH:mm");
return formatter.format(theDate);
}
public String getTitleDisplayText(DatePanel extends T> thePanel) {
final Date current = thePanel.getFirstDateShow();
final PanelType viewType = thePanel.getType();
switch (viewType) {
case MONTH: {
final Calendar helper = new GregorianCalendar();
helper.setMinimalDaysInFirstWeek(4);
helper.setFirstDayOfWeek(Calendar.MONDAY);
helper.setTime(current);
helper.add(Calendar.DATE, 14);
if (useShowMore()) {
final DateTimeFormat formatter = DateTimeFormat.getFormat("MMMM y"); // NOPMD;
return formatter.format(helper.getTime());
} else {
final DateTimeFormat formatter = DateTimeFormat.getFormat("MMMM yyyy"); // NOPMD;
return formatter.format(helper.getTime());
}
}
case WEEK: {
final Calendar helper = new GregorianCalendar();
helper.setMinimalDaysInFirstWeek(4);
helper.setFirstDayOfWeek(Calendar.MONDAY);
helper.setTime(current);
return dateTexts.week() + " " + (helper.get(Calendar.WEEK_OF_YEAR));
}
case DAY: {
DateTimeFormat formatter = DateTimeFormat.getFormat("MM/dd");
return formatter.format(current);
}
case LIST: {
DateTimeFormat formatter = DateTimeFormat.getFormat("MM/dd");
StringBuffer result = new StringBuffer();
result.append(formatter.format(current));
result.append(" - ");
final Date until = thePanel.getLastDateShow();
result.append(formatter.format(until));
return result.toString();
}
default: {
DateTimeFormat formatter = DateTimeFormat.getFormat("MM/dd");
return formatter.format(current);
}
}
}
public AddCallBackHandler createAddHandler(DatePanel view, T theData) {
return new AddCallBackHandler(view, theData);
}
public UpdateCallBackHandler createUpdateHandler(DatePanel view, T theData) {
return new UpdateCallBackHandler(view, theData);
}
public RemoveCallBackHandler createRemoveHandler(DatePanel view, T theData) {
return new RemoveCallBackHandler(view, theData);
}
public boolean showDay(int dayCode) {
return true;
}
public DateTimeFormat getDayHeaderDateTimeFormatter() {
return DateTimeFormat.getFormat("MM/dd E");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy