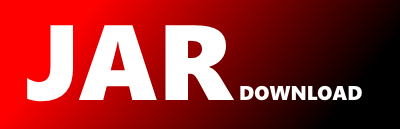
eu.future.earth.gwt.client.DateBoxInternal Maven / Gradle / Ivy
package eu.future.earth.gwt.client;
import java.util.Calendar;
import java.util.Date;
import com.google.gwt.i18n.client.DateTimeFormat;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.Event;
import com.google.gwt.user.client.ui.TextBox;
import eu.future.earth.gwt.client.date.DateUtils;
public class DateBoxInternal extends TextBox {
public final static String DEFAULT_DATE_FORMAT = "dd-MM-yyyy";
private String format = null;
private DateTimeFormat date = null;
public DateBoxInternal() {
this(DEFAULT_DATE_FORMAT);
}
public DateBoxInternal(String format) {
super();
super.sinkEvents(Event.ONKEYDOWN);
setFormat(format);
}
public void onBrowserEvent(Event event) {
int eventType = DOM.eventGetType(event);
int keyCode = event.getKeyCode();
switch (eventType) {
case Event.ONKEYDOWN: {
// if (keyCode == 37 || keyCode == 39 || keyCode == 18 || keyCode == 9 || keyCode == 8) {
if (keyCode == 37 || keyCode == 39 || keyCode == 18 || keyCode == 9) {
super.onBrowserEvent(event);
return;
} else {
if (isInt(keyCode)) {
final int cursor = super.getCursorPos();
if (cursor >= format.length()) {
} else {
final String temp = super.getText();
final char rep = format.charAt(cursor);
if (rep == '-' || rep == '/') {
super.setCursorPos(cursor + 1);
} else {
char next = (char) -1;
if (format.length() > cursor + 1) {
next = format.charAt(cursor + 1);
}
final char newChar = ((char) convert(keyCode));
boolean allow = false;
if (rep == 'd' && next == 'd') {
if (newChar == '0' || newChar == '1' || newChar == '2' || newChar == '3') {
allow = true;
}
} else {
if (rep == 'd') {
final char prev = temp.charAt(cursor - 1);
if (prev == '3') {
if (newChar == '0' || newChar == '1') {
allow = true;
}
} else {
allow = true;
}
}
}
if (rep == 'M' && next == 'M') {
if (newChar == '0' || newChar == '1') {
allow = true;
}
} else {
if (rep == 'M') {
final char prev = temp.charAt(cursor - 1);
if (prev == '0') {
allow = true;
} else {
if (newChar == '0' || newChar == '1' || newChar == '2') {
allow = true;
}
}
}
}
if (rep == 'y') {
allow = true;
}
if (allow) {
final String newString = temp.substring(0, cursor) + newChar + temp.substring(cursor + 1, format.length());
super.setText(newString);
if (next == '-' || rep == '/') {
super.setCursorPos(cursor + 2);
} else {
super.setCursorPos(cursor + 1);
}
}
}
}
event.preventDefault();
return;
} else {
if (keyCode == 8) {
final int cursor = super.getCursorPos() - 1;
if (cursor >= format.length() || cursor < 0) {
} else {
String sel = super.getSelectedText();
if (sel != null && sel.length() > 2) {
super.setText(format);
super.setCursorPos(0);
} else {
final String temp = super.getText();
final char rep = format.charAt(cursor);
final String newString = temp.substring(0, cursor) + rep + temp.substring(cursor + 1, format.length());
super.setText(newString);
super.setCursorPos(cursor);
}
}
event.preventDefault();
} else {
event.preventDefault();
return;
}
}
}
}
default:
super.onBrowserEvent(event);
}
}
private int convert(int keyCode) {
if (keyCode > 60) {
return keyCode - 48;
} else {
return keyCode;
}
}
private boolean isInt(int keyCode) {
if (keyCode >= 96 && keyCode <= 105) {
return true;
}
if (keyCode >= 48 && keyCode <= 57) {
return true;
}
return false;
}
public void setDate(Date newValue) {
if (newValue != null) {
super.setText(date.format(newValue));
} else {
super.setText(format);
}
}
private boolean isParsable() {
if (getText() == null) {
return false;
}
if (getText().length() != format.length()) {
return false;
}
return DateParser.testParsable(getText(), format);
}
public Date getDate() {
if (isParsable()) {
try {
Calendar helper = DateUtils.createCalendar();
helper.setTime(date.parse(getText()));
helper.add(Calendar.HOUR_OF_DAY, 12);
return helper.getTime();
} catch (IllegalArgumentException ex) {
return null;
} catch (Exception ex) {
return null;
}
}
return null;
}
public String getFormat() {
return format;
}
public void setFormat(String format) {
this.format = format;
date = DateTimeFormat.getFormat(format);
super.setText(format);
super.setMaxLength(format.length());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy