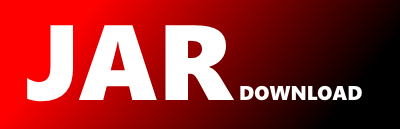
eu.future.earth.gwt.client.date.EventPanel Maven / Gradle / Ivy
/*
* Copyright 2007 Future Earth, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package eu.future.earth.gwt.client.date;
import java.util.Date;
import com.google.gwt.dom.client.Style.BorderStyle;
import com.google.gwt.dom.client.Style.Unit;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.user.client.ui.SimplePanel;
import com.google.gwt.user.client.ui.Widget;
public abstract class EventPanel extends SimplePanel implements HasDateEventHandlers, Comparable> {
private T value = null;
private TimeEventRenderer renderer = null;
@SuppressWarnings("unchecked")
protected EventPanel(TimeEventRenderer extends T> newRenderer, T newData) {
super();
renderer = (TimeEventRenderer) newRenderer;
value = newData;
setBackgroundColor("#F6F6F6");
}
public abstract void setContentHeight(int height);
public abstract int getContentHeight();
public abstract void setWidth(int width);
public int compareTo(EventPanel other) {
Date one = getStart();
Date two = other.getStart();
if (one != null) {
return one.compareTo(two);
}
return 0;
}
private String eventStyleName = null;
public String getEventStyleName() {
return eventStyleName;
}
public void setEventStyleName(String eventStyleName) {
this.eventStyleName = eventStyleName;
}
public void setBackgroundColor(String backgroundColor) {
if (backgroundColor == null) {
super.getElement().getStyle().clearBackgroundColor();
super.getElement().getStyle().clearBorderColor();
super.getElement().getStyle().clearBorderWidth();
super.getElement().getStyle().clearBorderStyle();
super.getElement().getStyle().clearColor();
} else {
super.getElement().getStyle().setBackgroundColor(backgroundColor);
super.getElement().getStyle().setBorderColor(CssColorHelper.determineBorderColor(backgroundColor));
super.getElement().getStyle().setBorderWidth(1, Unit.PX);
super.getElement().getStyle().setBorderStyle(BorderStyle.SOLID);
super.getElement().getStyle().setColor(CssColorHelper.determineFontColor(backgroundColor));
}
}
public void setStart(Date newStart) {
if (value != null) {
renderer.setStartTime(value, newStart);
}
}
public int getPosY() {
return posY;
}
public void setPosY(int posY) {
this.posY = posY;
}
private int posY = 0;
public void setEndTime(Date newEnd) {
if (value != null) {
renderer.setEndTime(value, newEnd);
}
}
public Date getStart() {
if (value != null) {
return renderer.getStartTime(value);
} else {
return null;
}
}
public Date getEnd() {
if (value != null) {
return renderer.getEndTime(value);
} else {
return null;
}
}
public T getValue() {
return value;
}
public void setValue(T value) {
this.value = value;
}
private HandlerRegistration parent = null;
public void addParentDateEventHandler(DateEventListener extends T> handler) {
parent = addDateEventHandler(handler);
}
public void clearParent() {
if (parent != null) {
parent.removeHandler();
}
}
public HandlerRegistration addDateEventHandler(DateEventListener extends T> handler) {
return addHandler(handler, DateEvent.getType());
}
/**
* Indicates whether some other object is "equal to" this one.
*
* @param obj
* the reference object with which to compare.
* @return true
if this object is the same as the obj argument; false
otherwise.
* @todo Implement this java.lang.Object method
*/
@SuppressWarnings("unchecked")
public boolean equals(Object obj) {
if (obj instanceof EventPanel>) {
final EventPanel extends T> real = (EventPanel extends T>) obj;
if (renderer == null) {
return value.equals(real.getValue());
}
if (renderer.equal(value, real.getValue())) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
return value.hashCode();
}
public boolean isDraggable() {
if (renderer == null) {
return false;
}
return renderer.enableDragAndDrop();
}
public abstract void repaintTime();
public abstract Widget getDraggableItem();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy