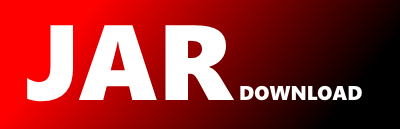
eu.future.earth.gwt.client.date.week.WeekPanel Maven / Gradle / Ivy
/*
* Copyright 2007 Future Earth, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package eu.future.earth.gwt.client.date.week;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.List;
import com.allen_sauer.gwt.dnd.client.drop.AbstractDropController;
import com.google.gwt.dom.client.Style.Unit;
import com.google.gwt.event.dom.client.ScrollEvent;
import com.google.gwt.event.dom.client.ScrollHandler;
import com.google.gwt.user.client.ui.AbsolutePanel;
import com.google.gwt.user.client.ui.DockLayoutPanel;
import com.google.gwt.user.client.ui.HTML;
import com.google.gwt.user.client.ui.ScrollPanel;
import eu.future.earth.gwt.client.FtrGwtDateCss;
import eu.future.earth.gwt.client.date.DatePanel;
import eu.future.earth.gwt.client.date.DateRenderer;
import eu.future.earth.gwt.client.date.DateUtils;
import eu.future.earth.gwt.client.date.EventPanel;
import eu.future.earth.gwt.client.date.PanelFactory;
import eu.future.earth.gwt.client.date.PanelType;
import eu.future.earth.gwt.client.date.WeekLabels;
import eu.future.earth.gwt.client.date.week.staend.AbstractDayField;
import eu.future.earth.gwt.client.date.week.staend.DayPanel;
import eu.future.earth.gwt.client.date.week.staend.DayPanelDropController;
import eu.future.earth.gwt.client.date.week.staend.HourPanel;
import eu.future.earth.gwt.client.date.week.staend.WeekPanelDragController;
import eu.future.earth.gwt.client.date.week.whole.DayPanelParent;
import eu.future.earth.gwt.client.date.week.whole.WholeDayDragController;
import eu.future.earth.gwt.client.date.week.whole.WholeDayPanelDropController;
public class WeekPanel extends BaseWeekPanel implements ScrollHandler, HasWeekScrollEventHandlers, DayPanelParent {
private AbsolutePanel body_drag = new AbsolutePanel();
private ScrollPanel body_scroller = new ScrollPanel(body_drag);
private AbsolutePanel wholeday_drag = new AbsolutePanel();
private ScrollPanel wholeday_scroll = new ScrollPanel(wholeday_drag);
private List dropControllers = new ArrayList();
private DockLayoutPanel main = new DockLayoutPanel(Unit.PX);
private WeekLabels main_labels = new WeekLabels();
private DockLayoutPanel main_whole_hor = new DockLayoutPanel(Unit.PX);
private DockLayoutPanel whole_days = new DockLayoutPanel(Unit.PCT);
private DockLayoutPanel main_body = new DockLayoutPanel(Unit.PX);
private DockLayoutPanel main_body_days = new DockLayoutPanel(Unit.PCT);
private DockLayoutPanel daysGrid = new DockLayoutPanel(Unit.PCT);
private WholeDayDragController wholeDayDragController = null;
private WeekPanelDragController weekDragController = null;
public WeekPanel(DateRenderer newRenderer) {
this(DateUtils.countDays(newRenderer), newRenderer);
}
public void scrollToHour(int hour) {
body_scroller.setVerticalScrollPosition(renderer.getIntervalHeight() * renderer.getIntervalsPerHour() * (hour - renderer.getStartHour()));
}
public void setSrolPos(int newPos) {
body_scroller.setVerticalScrollPosition(newPos);
}
public int getTopScrollable() {
return main_body.getAbsoluteTop();
}
public WeekPanel(int newNumberOfDays, DateRenderer newRenderer) {
this(newNumberOfDays, newRenderer, true);
}
public WeekPanel(int newNumberOfDays, DateRenderer newRenderer, boolean newShowDayLabel) {
super(newNumberOfDays, newRenderer, newShowDayLabel);
initWidget(main);
if (renderer.enableDragAndDrop()) {
weekDragController = new WeekPanelDragController(body_drag);
wholeDayDragController = new WholeDayDragController(wholeday_drag);
}
body_drag.add(main_body);
daysGrid.setStyleName(FtrGwtDateCss.WEEK_BODY);
buildPanel();
}
@SuppressWarnings("unchecked")
public void buildPanel() {
main.clear();
daysGrid.clear();
main_body_days.clear();
main_whole_hor.clear();
clearCache();
main_body.clear();
if (renderer.enableDragAndDrop()) {
for (AbstractDropController dropController : dropControllers) {
if (dropController instanceof DayPanelDropController>) {
final DayPanelDropController real = (DayPanelDropController) dropController;
weekDragController.unregisterDropController(real);
} else {
if (renderer.showWholeDayEventView()) {
if (dropController instanceof WholeDayPanelDropController>) {
final WholeDayPanelDropController real = (WholeDayPanelDropController) dropController;
wholeDayDragController.unregisterDropController(real);
}
}
}
}
dropControllers.clear();
}
HourPanel hour = new HourPanel(renderer);
if (isShowDayLabel() && daysShown > 1) {
main.addNorth(main_labels, 15);
main_labels.init(hour);
}
if (renderer.showWholeDayEventView()) {
main.addNorth(wholeday_scroll, renderer.getWholeEventRowHeigt());
wholeday_scroll.setStyleName(FtrGwtDateCss.WEEK_SCROLLER);
wholeday_scroll.addStyleName(FtrGwtDateCss.HEADER);
wholeday_drag.clear();
wholeday_drag.add(main_whole_hor);
main_whole_hor.addWest(new HTML(" "), hour.getPrefferedWitdhInt());
main_whole_hor.add(whole_days);
// CSS.setProperty(whole_days, CSS.A.BACKGROUND_COLOR, "#b6060a");
// whole_days.setStyleName(FtrGwtDateCss.WEEK_HEADER);
whole_days.clear();
wholeday_drag.setHeight(hour.getNeededHeight() + "px");
main_whole_hor.setHeight(hour.getNeededHeight() + "px");
// main_header_labels_days.setHeight(hour.getNeededHeight() + "px");
}
main.add(body_scroller);
body_scroller.setStyleName(FtrGwtDateCss.WEEK_SCROLLER);
main_body.setHeight(hour.getNeededHeight() + "px");
body_drag.setHeight(hour.getNeededHeight() + "px");
daysGrid.setHeight(hour.getNeededHeight() + "px");
main_body.addWest(hour, hour.getPrefferedWitdhInt());
// mainPanel.getCellFormatter().setWidth(0, 0, hour.getPrefferedWitdh());
// mainPanel.getCellFormatter().setStyleName(0, 0, FtrGwtDateCss.HOUR_MAIN_PANEL);
// mainPanel.getCellFormatter().setHorizontalAlignment(0, 0, HorizontalPanel.ALIGN_RIGHT);
Calendar helperCal = new GregorianCalendar();
helperCal.setTime(current.getTime());
helperCal.setFirstDayOfWeek(getFirstDayOfWeek());
if (daysShown > 1) {
helperCal.set(Calendar.DAY_OF_WEEK, getFirstDayOfWeek());
}
firstLogical = helperCal.getTime();
final Calendar today = new GregorianCalendar();
final int colWideProcent = (100 / daysShown);
for (int i = 0; i < daysShown; i++) {
int dayOfWeek = helperCal.get(Calendar.DAY_OF_WEEK);
if (renderer.showDay(dayOfWeek)) {
final Date currentDate = helperCal.getTime();
final DayPanel dayPanel = new DayPanel(renderer);
put(dayOfWeek, dayPanel);
if (DateUtils.isSameDay(today, helperCal)) {
dayPanel.addStyleName(FtrGwtDateCss.DATE_DAY_TODAY);
}
dayPanel.setDay(helperCal);
dayPanel.addDateEventHandler(this);
if (isShowDayLabel()) {
final DayHeader label = new DayHeader(currentDate, renderer);
put(dayOfWeek, label);
main_labels.addColumn(label, i, dayOfWeek, colWideProcent);
label.addDateEventHandler(this);
}
if (i == (daysShown - 1)) {
daysGrid.add(dayPanel);
} else {
daysGrid.addWest(dayPanel, colWideProcent);
}
if (renderer.enableDragAndDrop()) {
final DayPanelDropController dayController = new DayPanelDropController(dayPanel, this);
dropControllers.add(dayController);
weekDragController.registerDropController(dayController);
}
if (renderer.showWholeDayEventView()) {
final DayEventpanel eventPanel = new DayEventpanel(renderer, currentDate, this);
put(dayOfWeek, eventPanel);
if (i == (daysShown - 1)) {
whole_days.add(eventPanel);
} else {
whole_days.addWest(eventPanel, colWideProcent);
}
if (renderer.enableDragAndDrop()) {
final WholeDayPanelDropController dayController = new WholeDayPanelDropController(eventPanel);
dropControllers.add(dayController);
wholeDayDragController.registerDropController(dayController);
}
eventPanel.addDateEventHandler(this);
eventPanel.setDay(helperCal);
}
add(dayPanel);
} else {
i--;
}
helperCal.add(Calendar.DAY_OF_WEEK, 1);
}
main_body.add(daysGrid);
helperCal.add(Calendar.MINUTE, -1);
lastLogical = helperCal.getTime();
main.forceLayout();
main_body.forceLayout();
main_body_days.forceLayout();
daysGrid.forceLayout();
}
public PanelType getType() {
if (daysShown > 1) {
return PanelType.WEEK;
} else {
return PanelType.DAY;
}
}
@SuppressWarnings("unchecked")
public void createPanel(DayEventElement result, T newEvent, boolean partOfBatch) {
final EventPanel entry = (EventPanel) renderer.createPanel(newEvent, getType());
if (entry != null) {
if (entry instanceof AbstractDayField>) {
if (weekDragController != null && entry.isDraggable()) {
weekDragController.makeDraggable(entry, entry.getDraggableItem());
}
} else {
if (renderer.showWholeDayEventView()) {
if (wholeDayDragController != null && entry.isDraggable()) {
wholeDayDragController.makeDraggable(entry, entry.getDraggableItem());
}
}
}
result.addEvent(entry, partOfBatch);
entry.addParentDateEventHandler(this);
}
}
public void onScroll(ScrollEvent event) {
WeekScrollEvent.fire(this, body_scroller.getVerticalScrollPosition());
}
public static class FactoryWeekPanel implements PanelFactory {
public DatePanel createDatePanel(DateRenderer renderer) {
return new WeekPanel(DateUtils.countDays(renderer), renderer);
}
//Writing equals and hashCode methods such that various instances would appear as the same instance.
//Singleton could not be used due to use of generics.
public boolean equals(Object obj) {
return obj != null && getClass().equals( obj.getClass() );
}
public int hashCode() {
return getClass().hashCode();
}
}
public static class FactoryDayPanel implements PanelFactory {
public DatePanel createDatePanel(DateRenderer renderer) {
return new WeekPanel(1, renderer);
}
//Writing equals and hashCode methods such that various instances would appear as the same instance.
//Singleton could not be used due to use of generics.
public boolean equals(Object obj) {
return obj != null && getClass().equals( obj.getClass() );
}
public int hashCode() {
return getClass().hashCode();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy