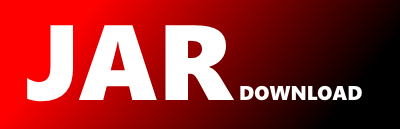
eu.future.earth.gwt.client.date.week.staend.DayPanelDropController Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2007 Future Earth, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package eu.future.earth.gwt.client.date.week.staend;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import com.allen_sauer.gwt.dnd.client.DragContext;
import com.allen_sauer.gwt.dnd.client.drop.SimpleDropController;
import com.google.gwt.user.client.ui.Widget;
import eu.future.earth.gwt.client.date.DateRenderer;
import eu.future.earth.gwt.client.date.DateUtils;
import eu.future.earth.gwt.client.date.week.WeekPanel;
/**
* DropController which allows a widget to be dropped on a SimplePanel drop target when the drop target does not yet
* have a child widget.
*/
public class DayPanelDropController extends SimpleDropController {
private Calendar helper = new GregorianCalendar(); // NOPMD;
private WeekPanel week = null;
public DayPanelDropController(DayPanel newDropTarget, WeekPanel newWeek) {
super(newDropTarget);
week = newWeek;
}
public void onDrop(DragContext context) {
final Widget drp = getDropTarget();
if (drp != null) {
final Widget drag = context.draggable;
if (drp instanceof DayPanel> && drag instanceof DayField>) {
@SuppressWarnings("unchecked")
final DayPanel parent = (DayPanel) drp;
@SuppressWarnings("unchecked")
final DayField data = (DayField) drag;
long duration = data.getEnd().getTime() - data.getStart().getTime();
DateRenderer renderer = parent.getRenderer();
int y = context.draggable.getAbsoluteTop() - week.getTopScrollable();
if (y < 0) {
y = 0;
}
y = y + (renderer.getStartHour() * renderer.getIntervalsPerHour() * renderer.getIntervalHeight());
helper.setTime(data.getStart());
// zero out time, keeping the date
helper.set(Calendar.HOUR_OF_DAY, 0);
helper.set(Calendar.MINUTE, 0);
helper.set(Calendar.SECOND, 0);
helper.set(Calendar.MILLISECOND, 0);
// now add however many minutes the nearest interval represents
helper.add(Calendar.MINUTE, (parent.calculateIntervalSnapForY(y)) * (60 / renderer.getIntervalsPerHour()));
Date dropOn = parent.getDate();
boolean sameDay = DateUtils.isSameDay(dropOn, data.getStart());
if (!sameDay) {
Calendar migrate = new GregorianCalendar(); // NOPMD;
migrate.setTime(dropOn);
helper.set(Calendar.YEAR, migrate.get(Calendar.YEAR));
helper.set(Calendar.MONTH, migrate.get(Calendar.MONTH));
helper.set(Calendar.DAY_OF_MONTH, migrate.get(Calendar.DAY_OF_MONTH));
}
data.setStart(helper.getTime());
data.setEndTime(new Date(data.getStart().getTime() + duration));
parent.addEventDrop(data, sameDay);
}
super.onDrop(context);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy