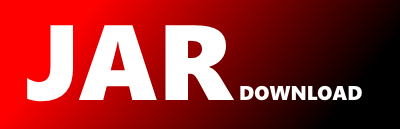
eu.future.earth.slider.client.SliderBarVertical Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2008 Google Inc. Licensed under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package eu.future.earth.slider.client;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.ClickHandler;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.event.dom.client.KeyUpEvent;
import com.google.gwt.event.dom.client.KeyUpHandler;
import com.google.gwt.event.dom.client.MouseWheelEvent;
import com.google.gwt.event.dom.client.MouseWheelHandler;
import com.google.gwt.event.logical.shared.HasValueChangeHandlers;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.event.logical.shared.ValueChangeHandler;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.Element;
import com.google.gwt.user.client.Event;
import com.google.gwt.user.client.ui.AbsolutePanel;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.FocusPanel;
import com.google.gwt.user.client.ui.HasValue;
import com.google.gwt.user.client.ui.Image;
import com.google.gwt.user.client.ui.Label;
import com.google.gwt.user.client.ui.RequiresResize;
import com.google.gwt.user.client.ui.SimplePanel;
/**
* A widget that allows the user to select a value within a range of possible values using a sliding bar that responds to mouse events. Keyboard
* Events
*
* SliderBarVertical listens for the following key events. Holding down a key will repeat the action until the key is released.
*
* - up arrow - shift up one step
* - down arrow - shift down one step
* - page up - shift up one page
* - page down - shift down one page
* - home - jump to min value
* - end - jump to max value
* - space - jump to middle value
*
*
*/
public class SliderBarVertical extends Composite implements RequiresResize, HasValue, HasValueChangeHandlers, BrowserEventParent {
public static final int DEFAULTPAGESIZE = 25;
/**
* The space bar key code.
*/
private static final int SPACEBAR = 32;
/**
* The current value.
*/
private int value;
/**
* A bit indicating whether or not the slider is enabled.
*/
private boolean enabled = true;
/**
* The images used with the sliding bar.
*/
private final SliderBarVerticalResources res;
/**
* The knob that slides across the line.
*/
private final Image knobImage;
/**
* The maximum slider value.
*/
private int maxValue;
/**
* The minimum slider value.
*/
private int minValue;
// private boolean snapToTick = false;
private int tickValue = 1;
private int majorTickValue = 5;
public int getMajorTickValue() {
return majorTickValue;
}
public void setMajorTickValue(int majorTickValue) {
this.majorTickValue = majorTickValue;
}
public int getTickValue() {
return tickValue;
}
public void setTickValue(int tickValue) {
this.tickValue = tickValue;
drawLabels();
}
/**
* A bit indicating whether or not we are currently sliding the slider bar due to mouse events.
*/
private boolean slidingMouse = false;
private AbsolutePanel main = new AbsolutePanel();
/**
* An empty constructor to support UI binder.
*
* @wbp.parser.constructor
*/
public SliderBarVertical() {
this(1, 1000, 1);
}
/**
* Create a slider bar.
*
* @param minValue2 the minimum value in the range
* @param maxValue2 the maximum value in the range
* @param pageSize2 the page size
*/
public SliderBarVertical(final int minValue2, final int maxValue2) {
this(minValue2, maxValue2, SliderBarVerticalResources.INSTANCE, minValue2);
}
public SliderBarVertical(final int minValue2, final int maxValue2, final int curValue2) {
this(minValue2, maxValue2, SliderBarVerticalResources.INSTANCE, curValue2);
}
private FocusPanel panel;
/**
* Create a slider bar.
*
* @param minValue2 the minimum value in the range
* @param maxValue2 the maximum value in the range
* @param images2 the images to use for the slider
* @param pageSize2 the page size
*/
public SliderBarVertical(final int minValue2, final int maxValue2, final SliderBarVerticalResources res2, final int curValue2) {
super();
res = res2;
res.sliderBarCss().ensureInjected();
minValue = minValue2;
maxValue = maxValue2;
value = curValue2;
panel = new SliderBarFocusPanel(this);
panel.setStyleName(res.sliderBarCss().sliderBarVerticalShell());
// Create the knob
knobImage = new Image(res.slider());
knobImage.setStyleName(res.sliderBarCss().sliderBarVerticalKnob());
panel.add(knobImage);
panel.sinkEvents(Event.MOUSEEVENTS | Event.KEYEVENTS | Event.FOCUSEVENTS | Event.ONMOUSEWHEEL);
panel.addKeyUpHandler(new KeyUpHandler() {
@Override
public void onKeyUp(KeyUpEvent event) {
if (enabled) {
doKeyAction(event.getNativeKeyCode());
}
}
});
panel.addClickHandler(new ClickHandler() {
@Override
public void onClick(ClickEvent event) {
if (enabled) {
setCurrentValue(getVlaueForY(event.getClientY()), true);
}
}
});
panel.addMouseWheelHandler(new MouseWheelHandler() {
@Override
public void onMouseWheel(MouseWheelEvent event) {
if (enabled) {
GWT.log("onmousewheel");
event.preventDefault();
final int deltaY = event.getDeltaY();
if (deltaY > 0) {
shiftDown(deltaY);
} else {
shiftUp(Math.abs(deltaY));
}
}
}
});
initWidget(main);
}
private int getVlaueForY(int y) {
if (y > 0) {
y -= 5;
final int lineTop = getAbsoluteTop();
final double percent = 1.0 - ((double) (y - lineTop) / getOffsetHeight() * 1.0);
return (int) (getTotalRange() * percent + minValue);
}
return value;
}
private boolean showLabels = true;
/**
* The value change handler.
*
* @param handler the handler
* @return the handle
*/
@Override
public final HandlerRegistration addValueChangeHandler(final ValueChangeHandler handler) {
return addHandler(handler, ValueChangeEvent.getType());
}
/**
* Allows access to the key events.
*
* @param keyCode
*/
public void doKeyAction(final int keyCode) {
switch (keyCode) {
case KeyCodes.KEY_HOME:
setCurrentValue(minValue);
break;
case KeyCodes.KEY_END:
setCurrentValue(maxValue);
break;
case KeyCodes.KEY_UP:
shiftUp(tickValue);
break;
case KeyCodes.KEY_DOWN:
shiftDown(tickValue);
break;
case SPACEBAR:
setCurrentValue((int) (minValue + getTotalRange() / 2));
break;
default:
}
}
private int labelSpacing = 15;
public int getLabelSpacing() {
return labelSpacing;
}
public void setLabelSpacing(int labelSpacing) {
this.labelSpacing = labelSpacing;
drawLabels();
}
private double getPosistionFor(int newValue) {
if (maxValue <= minValue) {
return 0;
}
final double percent = ((double) newValue - (double) minValue) / ((double) getTotalRange());
return (double) (height) - ((double) height * Math.max(0.0, Math.min(1.0, percent)));
}
private int height = 200;
private void drawLabels() {
if (isAttached()) {
main.clear();
height = (getTotalRange() * labelSpacing) / tickValue;
int newWidth = 20;
setPixelSize(newWidth + 30, height);
// GWT.log("Size = " + height);
if (showLabels) {
for (int i = minValue; i < maxValue;) {
i += tickValue;
double linePos = getPosistionFor(i);
SimplePanel line = new SimplePanel();
line.setPixelSize(newWidth, 1);
if (times(i, majorTickValue)) {
Label label = new Label(String.valueOf(i));
label.setHorizontalAlignment(Label.ALIGN_RIGHT);
main.add(label, newWidth + 2, (int) linePos);
line.setStyleName(res.sliderBarCss().majorLine());
} else {
line.setStyleName(res.sliderBarCss().minorLine());
}
main.add(line, 0, (int) linePos + 8);
}
}
main.add(panel, 0, 5);
panel.setPixelSize(newWidth, height);
}
}
/**
* Method Check's if the int Value is even or uneven.
*
* @param number - the integer to check.
* @param times - The number is has to be time to be a whole number when devided by.
* @return boolean - true if even false when uneven
*/
public static boolean times(int number, int times) {
if (number == 0) {
return false;
}
final double res = (double) number / (double) times;
final int test = (int) res;
return String.valueOf((double) test).equals(String.valueOf(res));
}
/**
* Draw the knob where it is supposed to be relative to the line.
*/
private void drawKnob() {
// Abort if not attached
if (!isAttached()) {
return;
}
// Move the knob to the correct position
final Element knobElement = knobImage.getElement();
final int knobTopOffset = (int) getPosistionFor(value);
DOM.setStyleAttribute(knobElement, "top", knobTopOffset -1 + "px");
}
/**
* Return the current value.
*
* @return the current value
*/
public final int getCurrentValue() {
return value;
}
/**
* Calculate the knob's position in coordinates.
*
* @return the knob's position
*/
private int getKnobPosition() {
return knobImage.getAbsoluteTop();
}
/**
* Return the max value.
*
* @return the max value
*/
public final double getMaxValue() {
return maxValue;
}
/**
* Return the minimum value.
*
* @return the minimum value
*/
public final double getMinValue() {
return minValue;
}
/**
* Return the total range between the minimum and maximum values.
*
* @return the total range
*/
public final int getTotalRange() {
if (minValue > maxValue) {
return 0;
} else {
return maxValue - minValue;
}
}
@Override
public final Integer getValue() {
return value;
}
/**
* @return Gets whether this widget is enabled
*/
public final boolean isEnabled() {
return enabled;
}
/**
* Listen for events that will move the knob.
*
* @param event the event that occurred
*/
@Override
public final void onBrowserFocusPanelEvent(final Event event) {
if (enabled) {
switch (DOM.eventGetType(event)) {
case Event.ONBLUR:
GWT.log("blur");
if (slidingMouse) {
slidingMouse = false;
slideKnob(event, true);
}
break;
// case Event.ONKEYDOWN:
// GWT.log("onkeydown");
// DOM.eventPreventDefault(event);
// if (slidingMouse) {
// slidingMouse = false;
// }
// doKeyAction(DOM.eventGetKeyCode(event));
// break;
// Mouse Events
case Event.ONMOUSEDOWN:
GWT.log("onmousedown");
if (sliderClicked(event)) {
slidingMouse = true;
DOM.setCapture(panel.getElement());
DOM.eventPreventDefault(event);
slideKnob(event, false);
}
break;
case Event.ONMOUSEUP:
GWT.log("onmouseup");
DOM.releaseCapture(panel.getElement());
if (slidingMouse) {
slideKnob(event, true);
slidingMouse = false;
}
break;
case Event.ONMOUSEMOVE:
if (slidingMouse) {
GWT.log("onmousemove");
slideKnob(event, false);
}
break;
default:
}
}
}
/**
* Slide the knob to a new location.
*
* @param event the mouse event
* @param fireEvent whether or not to just slide the knob
*/
private void slideKnob(final Event event, final boolean fireEvent) {
final int y = DOM.eventGetClientY(event);
if (y > 0) {
setCurrentValue(getVlaueForY(y), fireEvent);
}
}
/**
* Checks whether the slider was clicked or not.
*
* @param event the mouse event
* @return whether the slider was clicked
*/
private boolean sliderClicked(final Event event) {
boolean sliderClicked = false;
final int y = DOM.eventGetClientY(event);
final int knopPos = getKnobPosition();
GWT.log("y = " + y + " and pos = " + knopPos);
if (y < (knopPos + 7) && y > (knopPos - 7)) {
// if (DOM.eventGetTarget(event).equals(knobImage.getElement())) {
sliderClicked = true;
GWT.log("treuueeeeee ");
}
return sliderClicked;
}
/**
* Handle the resize event.
*/
@Override
public void onResize() {
if (isAttached()) {
drawKnob();
}
}
/**
* Reset the progress to constrain the progress to the current range and redraw the knob as needed.
*
* @param fireEvent
*/
private void resetCurrentValue(final boolean fireEvent) {
setCurrentValue(getCurrentValue(), fireEvent);
}
/**
* Set the current value and fire the onValueChange event.
*
* @param curValue2 the current value
*/
public final void setCurrentValue(final int curValue2) {
setCurrentValue(curValue2, true);
}
/**
* Set the current value and optionally fire the onValueChange event.
*
* @param curValue2 the current value
* @param fireEvent fire the onValue change event if true
*/
public final void setCurrentValue(final int newValue, final boolean fireEvent) {
// Confine the value to the range
GWT.log("new = " + newValue);
int curValue2 = snapToTickValue(newValue);
value = Math.max(minValue, Math.min(maxValue, curValue2));
// Redraw the knob
drawKnob();
GWT.log("cu" + curValue2);
// Fire the ValueChangeEvent
if (fireEvent) {
// keyTimer.cancel();
ValueChangeEvent.fire(this, value);
}
}
public int snapToTickValue(int y) {
if (tickValue == 1) {
return y;
}
return (int) (Math.round(((double) y) / tickValue) * tickValue);
}
@Override
protected void onAttach() {
super.onAttach();
drawLabels();
drawKnob();
}
/**
* Sets whether this widget is enabled.
*
* @param enabled2 true to enable the widget, false to disable it
*/
public final void setEnabled(final boolean enabled2) {
enabled = enabled2;
if (enabled) {
knobImage.removeStyleName(res.sliderBarCss().sliderDisabled());
} else {
knobImage.addStyleName(res.sliderBarCss().sliderDisabled());
}
}
/**
* Set the max value.
*
* @param maxValue2 the max value
* @param fireEvent
*/
public final void setMaxValue(final int maxValue2) {
maxValue = maxValue2;
resetCurrentValue(true);
drawLabels();
}
/**
* Set the max value.
*
* @param maxValue2 the max value
* @param fireEvent
*/
public final void setMaxValue(final int maxValue2, final boolean fireEvent) {
maxValue = maxValue2;
resetCurrentValue(fireEvent);
}
/**
* Set the minimum value.
*
* @param minValue2 the current value
*/
public final void setMinValue(final int minValue2) {
minValue = minValue2;
resetCurrentValue(true);
drawLabels();
}
/**
* Set the value of the slider in real terms.
*
* @param value the value
*/
@Override
public final void setValue(final Integer value) {
setCurrentValue(value, false);
}
/**
* Set the value of the slider in real terms.
*
* @param value the value
* @param fireEvent whether to fire an event
*/
@Override
public final void setValue(final Integer value, final boolean fireEvent) {
setCurrentValue(value, fireEvent);
}
/**
* Shift to the right (greater value).
*
* @param numSteps the number of steps to shift
*/
public final void shiftDown(final int numSteps) {
setCurrentValue(getCurrentValue() - numSteps);
}
/**
* Shift to the left (smaller value).
*
* @param numSteps the number of steps to shift
*/
public final void shiftUp(final int numSteps) {
setCurrentValue(getCurrentValue() + numSteps);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy