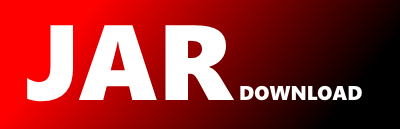
com.google.gwt.user.client.ui.CleanRadioButton Maven / Gradle / Ivy
The newest version!
package com.google.gwt.user.client.ui;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.i18n.shared.DirectionEstimator;
import com.google.gwt.uibinder.client.UiConstructor;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.Event;
/**
* A clearn radio button without vrappers.
* @author mnouwens
*
*/
public class CleanRadioButton extends CleanCheckBox {
public static final DirectionEstimator DEFAULT_DIRECTION_ESTIMATOR = DirectionalTextHelper.DEFAULT_DIRECTION_ESTIMATOR;
private Boolean oldValue;
/**
* Creates a new radio associated with a particular group name. All radio buttons associated with the same group
* name belong to a mutually-exclusive set.
*
* Radio buttons are grouped by their name attribute, so changing their name using the setName() method will also
* change their associated group.
*
* @param name
* the group name with which to associate the radio button
*/
@UiConstructor
public CleanRadioButton(String name) {
super(DOM.createInputRadio(name));
sinkEvents(Event.ONCLICK);
sinkEvents(Event.ONMOUSEUP);
sinkEvents(Event.ONBLUR);
sinkEvents(Event.ONKEYDOWN);
}
/**
* Overridden to send ValueChangeEvents only when appropriate.
*/
@Override
public void onBrowserEvent(Event event) {
switch (DOM.eventGetType(event)) {
case Event.ONMOUSEUP:
case Event.ONBLUR:
case Event.ONKEYDOWN:
// Note the old value for onValueChange purposes (in ONCLICK case)
oldValue = getValue();
break;
case Event.ONCLICK:
// It's not the label. Let our handlers hear about the
// click...
super.onBrowserEvent(event);
ValueChangeEvent.fireIfNotEqual(CleanRadioButton.this, oldValue, getValue());
return;
}
super.onBrowserEvent(event);
}
/**
* Change the group name of this radio button.
*
* Radio buttons are grouped by their name attribute, so changing their name using the setName() method will also
* change their associated group.
*
* If changing this group name results in a new radio group with multiple radio buttons selected, this radio button
* will remain selected and the other radio buttons will be unselected.
*
* @param name
* name the group with which to associate the radio button
*/
@Override
public void setName(String name) {
// Just changing the radio button name tends to break groupiness,
// so we have to replace it. Note that replaceInputElement is careful
// not to propagate name when it propagates everything else
replaceInputElement(DOM.createInputRadio(name));
}
@Override
public void sinkEvents(int eventBitsToAdd) {
// Like CheckBox, we want to hear about inputElem. We
// also want to know what's going on with the label, to
// make sure onBrowserEvent is able to record value changes
// initiated by label events
if (isOrWasAttached()) {
Event.sinkEvents(inputElem, eventBitsToAdd | Event.getEventsSunk(inputElem));
// Event.sinkEvents(labelElem, eventBitsToAdd | Event.getEventsSunk(labelElem));
} else {
super.sinkEvents(eventBitsToAdd);
}
}
/**
* No-op. CheckBox's click handler is no good for radio button, so don't use it. Our event handling is all done in
* {@link #onBrowserEvent}
*/
@Override
protected void ensureDomEventHandlers() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy