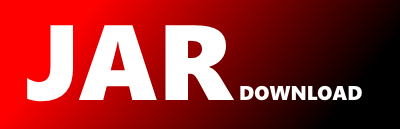
eu.future.earth.gwt.client.ui.actionevent.ActionEvent Maven / Gradle / Ivy
The newest version!
package eu.future.earth.gwt.client.ui.actionevent;
import com.google.gwt.event.shared.GwtEvent;
public class ActionEvent extends GwtEvent> {
/**
* Handler type.
*/
private static Type> TYPE;
/**
* Fires a before selection event on all registered handlers in the handler manager. If no such handlers exist, this
* method will do nothing.
*
* @param source
* the source of the handlers
* @return the event so that the caller can check if it was canceled, or null if no handlers of this event type have
* been registered
*/
public static ActionEvent fire(HasActionHandlers source, T newValue) {
// If no handlers exist, then type can be null.
if (TYPE != null) {
final ActionEvent event = new ActionEvent(newValue);
source.fireEvent(event);
return event;
}
return null;
}
/**
* Convenience method to allow subtypes to know when they should fire a value change event in a null-safe manner.
*
* @param source
* the source
* @param oldValue
* the old value
* @param newValue
* the new value
* @return whether the event should be fired
*/
protected static boolean shouldFire(HasActionHandlers source, T oldValue, T newValue) {
return TYPE != null && oldValue != newValue && (oldValue == null || !oldValue.equals(newValue));
}
/**
* Fires value change event if the old value is not equal to the new value. Use this call rather than making the
* decision to short circuit yourself for safe handling of null.
*
* @param source
* the source of the handlers
* @param oldValue
* the oldValue, may be null
* @param newValue
* the newValue, may be null
*/
public static void fireIfNotEqual(HasActionHandlers source, T oldValue, T newValue) {
if (shouldFire(source, oldValue, newValue)) {
ActionEvent event = new ActionEvent(newValue);
source.fireEvent(event);
}
}
/**
* Gets the type associated with this event.
*
* @return returns the handler type
*/
public static Type> getType() {
if (TYPE == null) {
TYPE = new Type>();
}
return TYPE;
}
protected ActionEvent(T newValue) {
super();
value = newValue;
}
private final T value;
public T getValue() {
return value;
}
@SuppressWarnings({
"unchecked", "rawtypes"
})
@Override
public final Type> getAssociatedType() {
return (Type) TYPE;
}
@Override
protected void dispatch(ActionHandler handler) {
handler.onKeyChange(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy