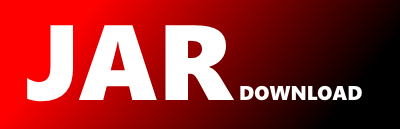
eu.future.earth.gwt.client.ui.button.ImageAnchor Maven / Gradle / Ivy
The newest version!
package eu.future.earth.gwt.client.ui.button;
import com.google.gwt.dom.client.Element;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.ClickHandler;
import com.google.gwt.event.dom.client.HasClickHandlers;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.uibinder.client.UiConstructor;
import com.google.gwt.user.client.Event;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.FocusPanel;
import com.google.gwt.user.client.ui.Focusable;
import com.google.gwt.user.client.ui.Image;
import com.google.gwt.user.client.ui.InlineLabel;
import eu.future.earth.gwt.client.ui.event.ClickTouchEvent;
import eu.future.earth.gwt.client.ui.event.ClickTouchHandler;
import eu.future.earth.gwt.client.ui.event.HasClickTouchHandlers;
public class ImageAnchor extends Composite implements HasClickHandlers, Focusable, HasClickTouchHandlers {
protected FocusPanel main = new FocusPanel();
protected InlineLabel text = new InlineLabel();
@UiConstructor
public ImageAnchor() {
this(null, null, UiType.Touch);
}
public ImageAnchor(UiType forTouch) {
this(null, null, forTouch);
}
public ImageAnchor(String newText) {
this(newText, null, UiType.Touch);
}
public ImageAnchor(String newText, UiType forTouch) {
this(newText, null, forTouch);
}
public ImageAnchor(Image newImage) {
this(null, newImage);
}
public ImageAnchor(Image newImage, UiType forTouch) {
this(null, newImage, forTouch);
}
public ImageAnchor(String newText, Image newImage) {
this(newText, newImage, UiType.Touch);
}
public ImageAnchor(String newText, Image newImage, UiType forTouch) {
this(newText, newImage, forTouch, true);
}
public ImageAnchor(String newText, Image newImage, UiType forTouch, boolean setTouch) {
super();
initWidget(main);
super.addStyleName("ftr-image-anchor");
if (newImage == null || setTouch) {
if (UiType.Touch.equals(forTouch)) {
super.addStyleName("touch");
} else {
super.addStyleName("notouch");
}
}
main.addClickHandler(new ClickHandler() {
@Override
public void onClick(ClickEvent event) {
if (enabled) {
handleClick();
}
}
});
setImage(newImage);
if (newText != null) {
main.getElement().appendChild(text.getElement());
text.setText(newText);
}
}
public void handleClick() {
ClickTouchEvent.fire(this);
}
public void addStyleNameToMain(String newStyle) {
main.addStyleName(newStyle);
}
protected void removeStyleNameToMain(String newStyle) {
main.removeStyleName(newStyle);
}
private Element imagePlaced = null;
public void setImage(Image newImage) {
if (imagePlaced != null) {
main.getElement().removeChild(imagePlaced);
}
if (newImage != null) {
imagePlaced = newImage.getElement();
main.getElement().insertFirst(imagePlaced);
}
}
public void setText(String newText) {
text.setText(newText);
if (!main.getElement().isOrHasChild(text.getElement())) {
main.getElement().appendChild(text.getElement());
}
}
private boolean enabled = true;
public void setEnabled(boolean newState) {
if (enabled != newState) {
if (enabled) {
super.addStyleName("ftr-disabled");
} else {
super.removeStyleName("ftr-disabled");
}
enabled = newState;
}
}
public boolean isEnabled() {
return enabled;
}
@Override
public void onBrowserEvent(Event event) {
if (enabled) {
// if (DOM.eventGetType(event) == Event.ONCLICK) {
//
// }
super.onBrowserEvent(event);
// handleClick();
} else {
event.preventDefault();
}
}
@Override
public HandlerRegistration addClickHandler(ClickHandler handler) {
return addHandler(handler, ClickEvent.getType());
}
@Override
public int getTabIndex() {
return main.getTabIndex();
}
@Override
public void setAccessKey(char key) {
main.setAccessKey(key);
}
@Override
public void setFocus(boolean focused) {
main.setFocus(focused);
}
@Override
public void setTabIndex(int index) {
main.setTabIndex(index);
}
@Override
public HandlerRegistration addClickHandler(ClickTouchHandler handler) {
return super.addHandler(handler, ClickTouchEvent.getType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy