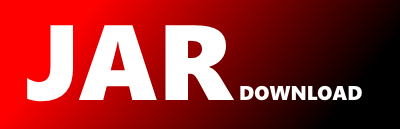
eu.future.earth.gwt.client.ui.general.CssHelper Maven / Gradle / Ivy
package eu.future.earth.gwt.client.ui.general;
import com.google.gwt.dom.client.Element;
import com.google.gwt.dom.client.Style.BorderStyle;
import com.google.gwt.user.client.ui.Widget;
public class CssHelper {
/**
* This class contains all CSS Attributes.
*/
public static final class A {
/**
* BACKGROUND_ATTACHMENT
*
* CSS: 'background-attachment', javaScript: 'backgroundAttachment'
* Values: scroll | fixed | inherit
* Initial value: scroll
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BACKGROUND_ATTACHMENT = "backgroundAttachment";
/**
* BACKGROUND_COLOR
*
* CSS: 'background-color', javaScript: 'backgroundColor'
* Values: <color> | transparent | inherit
* Initial value: transparent
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BACKGROUND_COLOR = "backgroundColor";
/**
* BACKGROUND_IMAGE
*
* CSS: 'background-image', javaScript: 'backgroundImage'
* Values: <uri> | none | inherit
* Initial value: none
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BACKGROUND_IMAGE = "backgroundImage";
/**
* BACKGROUND_POSITION
*
* CSS: 'background-position', javaScript: 'backgroundPosition'
* Values: [ [ <percentage> | <length> | left | center | right ] [ <percentage> |
* <length> | top | center | bottom ]? ] | [ [ left | center | right ] || [ top | center | bottom ] ] |
* inherit
* Initial value: 0% 0%
* Applies to: all
* Inherited?: no
* Percentages: refer to the size of the box itself
* Media groups: visual
*/
public static final String BACKGROUND_POSITION = "backgroundPosition";
/**
* BACKGROUND_REPEAT
*
* CSS: 'background-repeat', javaScript: 'backgroundRepeat'
* Values: repeat | repeat-x | repeat-y | no-repeat | inherit
* Initial value: repeat
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BACKGROUND_REPEAT = "backgroundRepeat";
/**
* BORDER_TOP
*
* CSS: 'border-top', javaScript: 'borderTop'
* Values: [ <border-width> || <border-style> || 'border-top-color' ] | inherit
* Initial value: see individual properties
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_TOP = "borderTop";
/**
* BORDER_RIGHT
*
* CSS: 'border-right', javaScript: 'borderRight'
* Values: [ <border-width> || <border-style> || 'border-top-color' ] | inherit
* Initial value: see individual properties
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_RIGHT = "borderRight";
/**
* BORDER_BOTTOM
*
* CSS: 'border-bottom', javaScript: 'borderBottom'
* Values: [ <border-width> || <border-style> || 'border-top-color' ] | inherit
* Initial value: see individual properties
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_BOTTOM = "borderBottom";
/**
* BORDER_LEFT
*
* CSS: 'border-left', javaScript: 'borderLeft'
* Values: [ <border-width> || <border-style> || 'border-top-color' ] | inherit
* Initial value: see individual properties
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_LEFT = "borderLeft";
/**
* BORDER_TOP_COLOR
*
* CSS: 'border-top-color', javaScript: 'borderTopColor'
* Values: <color> | transparent | inherit
* Initial value: the value of the 'color' property
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_TOP_COLOR = "borderTopColor";
/**
* BORDER_RIGHT_COLOR
*
* CSS: 'border-right-color', javaScript: 'borderRightColor'
* Values: <color> | transparent | inherit
* Initial value: the value of the 'color' property
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_RIGHT_COLOR = "borderRightColor";
/**
* BORDER_BOTTOM_COLOR
*
* CSS: 'border-bottom-color', javaScript: 'borderBottomColor'
* Values: <color> | transparent | inherit
* Initial value: the value of the 'color' property
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_BOTTOM_COLOR = "borderBottomColor";
/**
* BORDER_LEFT_COLOR
*
* CSS: 'border-left-color', javaScript: 'borderLeftColor'
* Values: <color> | transparent | inherit
* Initial value: the value of the 'color' property
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_LEFT_COLOR = "borderLeftColor";
/**
* BORDER_TOP_STYLE
*
* CSS: 'border-top-style', javaScript: 'borderTopStyle'
* Values: <border-style> | inherit
* Initial value: none
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_TOP_STYLE = "borderTopStyle";
/**
* BORDER_RIGHT_STYLE
*
* CSS: 'border-right-style', javaScript: 'borderRightStyle'
* Values: <border-style> | inherit
* Initial value: none
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_RIGHT_STYLE = "borderRightStyle";
/**
* BORDER_BOTTOM_STYLE
*
* CSS: 'border-bottom-style', javaScript: 'borderBottomStyle'
* Values: <border-style> | inherit
* Initial value: none
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_BOTTOM_STYLE = "borderBottomStyle";
/**
* BORDER_LEFT_STYLE
*
* CSS: 'border-left-style'', javaScript: 'borderLeftStyle'
* Values: <border-style> | inherit
* Initial value: none
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_LEFT_STYLE = "borderLeftStyle";
/**
* BORDER_TOP_WIDTH
*
* CSS: 'border-top-width', javaScript: 'borderTopWidth'
* Values: <border-width> | inherit
* Initial value: medium
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_TOP_WIDTH = "borderTopWidth";
/**
* BORDER_RIGHT_WIDTH
*
* CSS: 'border-right-width', javaScript: 'borderRightWidth'
* Values: <border-width> | inherit
* Initial value: medium
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_RIGHT_WIDTH = "borderRightWidth";
/**
* BORDER_BOTTOM_WIDTH
*
* CSS: 'border-bottom-width, javaScript: 'borderBottomWidth'
* Values: <border-width> | inherit
* Initial value: medium
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_BOTTOM_WIDTH = "borderBottomWidth";
/**
* BORDER_LEFT_WIDTH
*
* CSS: 'border-left-width', javaScript: 'borderLeftWidth'
* Values: <border-width> | inherit
* Initial value: medium
* Applies to: all
* Inherited?: no
* Media groups: visual
*/
public static final String BORDER_LEFT_WIDTH = "borderLeftWidth";
}
public static final class V {
public static final class BORDER_WIDTH {
public static final String THIN = "1px";
}
public static final class FONT_SIZE {
public static final double X_LARGE = 22;
}
/**
* CSS property {@link A#BACKGROUND_POSITION} values.
*/
public static final class BACKGROUND_POSITION {
public static final String LEFT = "left";
public static final String CENTER = "center";
public static final String RIGHT = "right";
public static final String TOP = "top";
}
/**
* CSS property {@link A#BACKGROUND_REPEAT} values.
*/
public static final class BACKGROUND_REPEAT {
/**
* Default value for {@link A#BACKGROUND_REPEAT }.
*/
public static final String REPEAT = "repeat";
public static final String REPEAT_X = "repeat-x";
public static final String REPEAT_Y = "repeat-y";
public static final String NO_REPEAT = "no-repeat";
}
}
/**
* Convenience method to set a style property on a widget.
*
*
* The GWT compiler will optimize this method away, meaning there are no additional costs of an extra method call
* when using method.
*
* @param widget
* Widget to set the property on
* @param name
* Name of the property
* @param value
* Value of the property
*/
public static void setProperty(Widget widget, String name, String value) {
widget.getElement().getStyle().setProperty(name, value);
}
public static void setProperty(Element widget, String name, String value) {
widget.getStyle().setProperty(name, value);
}
public static void setBorderBottumColor(Widget fits, String color) {
setBorderBottumColor(fits, 3, color);
}
public static void setBorderBottumColor(Widget fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_WIDTH, "0px");
}
}
public static void setBorderBottumColor(Element fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_BOTTOM_WIDTH, "0px");
}
}
public static void setBorderRightColor(Element fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_RIGHT_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_RIGHT_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_RIGHT_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_RIGHT_WIDTH, "0px");
}
}
public static void setBorderLeftColor(Element fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_LEFT_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_LEFT_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_LEFT_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_LEFT_WIDTH, "0px");
}
}
public static void setBorderTopColor(Widget fits, String color) {
setBorderTopColor(fits, 3, color);
}
public static void setBorderTopColor(Widget fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_WIDTH, "0px");
}
}
public static void setBorderTopColor(Element fits, int wide, String color) {
if (color != null) {
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_COLOR, color);
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_STYLE, BorderStyle.SOLID.getCssName());
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_WIDTH, wide + "px");
} else {
CssHelper.setProperty(fits, CssHelper.A.BORDER_TOP_WIDTH, "0px");
}
}
public static void setCornerToRed(Widget fits, String color) {
fits.addStyleName("creche-no-picture");
}
public static void setBottomCornerToRed(Widget fits, String color) {
fits.addStyleName("creche-play-outside");
}
}