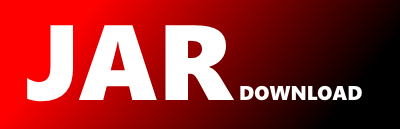
eu.future.earth.gwt.client.ui.general.WindowUtils Maven / Gradle / Ivy
The newest version!
package eu.future.earth.gwt.client.ui.general;
import java.util.Date;
public class WindowUtils {
public static void gotoFullScreen() {
toFullScreen();
}
public static native void toFullScreen() /*-{
var docElm = $doc.documentElement;
if (docElm.requestFullscreen) {
docElm.requestFullscreen();
} else if (docElm.mozRequestFullScreen) {
docElm.mozRequestFullScreen();
} else if (docElm.webkitRequestFullscreen) {
docElm.webkitRequestFullscreen ();
}
}-*/;
public static native boolean testAllowsFullscreen() /*-{
var docElm = $doc.documentElement;
if (docElm.requestFullscreen) {
return true;
} else if (docElm.mozRequestFullScreen) {
return true;
} else if (docElm.webkitRequestFullScreen) {
return true;
}
return false;
}-*/;
public static void cancelFullScreen() {
closeFullScreen();
}
public static native void closeFullScreen() /*-{
if ($doc.exitFullscreen) {
$doc.exitFullscreen();
} else if ($doc.mozCancelFullScreen) {
$doc.mozCancelFullScreen();
} else if ($doc.webkitCancelFullScreen) {
$doc.webkitCancelFullScreen();
}
}-*/;
public static boolean allowsFullScreen() {
return testAllowsFullscreen();
}
public static String createUrl(String string, String newHistoryToken) {
String newBase = string;
if (string.contains("#")) {
newBase = string.substring(0, string.indexOf("#") + 1);
}
return newBase + newHistoryToken;
}
public static String getStringParameter(String parameterName) {
String reservationStringId = com.google.gwt.user.client.Window.Location.getParameter(parameterName);
if (reservationStringId != null && reservationStringId.length() > 0) {
return reservationStringId;
}
return null;
}
public static long getLongParameter(String parameterName) {
String reservationStringId = com.google.gwt.user.client.Window.Location.getParameter(parameterName);
if (reservationStringId != null && reservationStringId.length() > 0) {
return Long.parseLong(reservationStringId);
}
return -1;
}
public static boolean getBooleanParameter(String parameterName) {
String reservationStringId = com.google.gwt.user.client.Window.Location.getParameter(parameterName);
if (reservationStringId != null && reservationStringId.length() > 0) {
return Boolean.parseBoolean(reservationStringId);
}
return false;
}
public static Date getDateParameter(String parameterName) {
String reservationStringId = com.google.gwt.user.client.Window.Location.getParameter(parameterName);
if (reservationStringId != null && reservationStringId.length() > 0) {
return new Date(Long.parseLong(reservationStringId));
}
return null;
}
public static void goToUrl(String website) {
changeUrl(website);
}
public static void sendToAdressAndBreakout(String website) {
if (website != null && website.length() > 0) {
if (website.contains("http")) {
changeUrlAndBreakOut(website);
} else {
changeUrlAndBreakOut("http://" + website);
}
} else {
changeUrlAndBreakOut("/");
}
}
public static void sendToAdressNoBreakOut(String website) {
if (website != null && website.length() > 0) {
if (website.contains("http")) {
changeUrl(website);
} else {
changeUrl("http://" + website);
}
} else {
changeUrl("/");
}
}
protected static native void changeUrl(String url)
/*-{
$wnd.location.href = url;
}-*/
;
protected static native void changeUrlAndBreakOut(String url)
/*-{
$wnd.top.location = url;
}-*/
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy