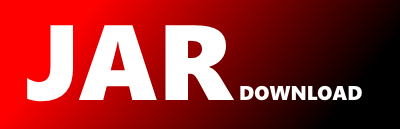
eu.ginere.base.util.image.ImageUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ginere-base Show documentation
Show all versions of ginere-base Show documentation
Base utilities for java projects
/**
* Copyright: Angel-Ventura Mendo Gomez
* [email protected]
*
* $Id: ImageUtils.java,v 1.1 2006/11/25 07:24:14 ventura Exp $
*/
package eu.ginere.base.util.image;
import eu.ginere.base.util.dao.DaoManagerException;
import eu.ginere.base.util.file.FileConnector;
import eu.ginere.base.util.file.FileConnectorInterface;
import eu.ginere.base.util.file.FileId;
import eu.ginere.base.util.file.FileInfo;
import eu.ginere.base.util.image.ImageSize.Dimension;
import eu.ginere.base.util.image.ImageSize.FormatNotSuported;
import eu.ginere.base.util.image.ImageSize.ImageSizeException;
import java.awt.AWTException;
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import java.awt.image.AffineTransformOp;
import java.awt.image.BufferedImage;
import java.awt.image.ColorModel;
import java.awt.image.IndexColorModel;
import java.awt.image.Raster;
import java.awt.image.WritableRaster;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.imageio.ImageIO;
import org.apache.commons.io.IOUtils;
import org.apache.log4j.Logger;
import com.sun.image.codec.jpeg.JPEGCodec;
import com.sun.image.codec.jpeg.JPEGEncodeParam;
import com.sun.image.codec.jpeg.JPEGImageEncoder;
/*
* This is for images stored into the system as files
*
* @author Angel Mendo
* @version $Revision: 1.1 $
*/
public class ImageUtils {
private static Logger log = Logger.getLogger(ImageUtils.class);
protected static final float JPEG_QUALITY=(float)0.6;
// /**
// * Uses the oldThumbnail as a file if possible
// */
// public static FileId updateOrCreateThumbnail(FileId oldThumbnail,FileId image,int maxWidth,int maxHeight)throws DaoManagerException,IOException{
// if (!ImageSize.isImage(image)){
// return null;
// } else {
// try {
// int newWidth=ImageSize.getResizedWidth(image,maxWidth,maxHeight);
// int newHeight=ImageSize.getResizedHeight(image,maxWidth,maxHeight);
//
// BufferedImage buffer=createImage(image);
// BufferedImage result=scaleImage(buffer,newWidth,newHeight);
// return updateImage(oldThumbnail,image,result);
// }catch (ImageSizeException e) {
// throw new DaoManagerException("FileName:"+image, e);
// }catch (FormatNotSuported e) {
// throw new DaoManagerException("FileName:"+image, e);
// }
// }
// }
// /**
// *
// * @param image
// * @param maxWidth
// * @param maxHeight
// * @return null if the thumbnail can't be created
// * @throws FileManagerException
// * @throws IOException
// */
// public static FileId createThumbnail(FileId image,int maxWidth,int maxHeight)throws DaoManagerException,IOException{
// return updateOrCreateThumbnail(null,image,maxWidth,maxWidth);
// }
// /**
// * Resize the image into this file, if any
// * @param file The file representing the image to transform
// * @param width maxWidth, maxHeight
// * @param height
// */
// public static void resizeImage(FileId image,int maxWidth,int maxHeight)throws DaoManagerException,IOException{
// if (!ImageSize.isImage(image)){
// return ;
// } else {
// try {
// int newWidth=ImageSize.getResizedWidth(image,maxWidth,maxHeight);
// int newHeight=ImageSize.getResizedHeight(image,maxWidth,maxHeight);
//
// BufferedImage buffer=createImage(image);
// BufferedImage result=scaleImage(buffer,newWidth,newHeight);
// writeImage(image,result);
// }catch (ImageSizeException e) {
// throw new DaoManagerException("FileName:"+image, e);
// }catch (FormatNotSuported e) {
// throw new DaoManagerException("FileName:"+image, e);
// }
// }
// }
/**
* Resize the image into this file, if any
* @param file The file representing the image to transform
* @param width maxWidth, maxHeight
* @param height
*/
public static FileId createImage(String fileName,String mimeType,String userId,File file,int maxWidth,int maxHeight)throws DaoManagerException,IOException{
if (!ImageSize.isImage(mimeType)){
return null;
} else {
try {
Dimension d = ImageSize.getDimensions(fileName,mimeType,file);
int newWidth=ImageSize.getResizedWidth(d,maxWidth,maxHeight);
int newHeight=ImageSize.getResizedHeight(d,maxWidth,maxHeight);
FileInputStream input=new FileInputStream(file);
try {
BufferedImage buffer=createImage(input);
BufferedImage result=scaleImage(buffer,newWidth,newHeight);
return createImage(fileName, mimeType, userId, result);
}finally{
input.close();
}
}catch (ImageSizeException e) {
throw new DaoManagerException("FileName:"+fileName, e);
}catch (FormatNotSuported e) {
throw new DaoManagerException("FileName:"+fileName, e);
}
}
}
public static FileId createImage(String fileName,String mimeType,String userId,byte bytes[],int maxWidth,int maxHeight)throws DaoManagerException,IOException{
if (!ImageSize.isImage(mimeType)){
return null;
} else {
try {
ByteArrayInputStream inputDim=new ByteArrayInputStream(bytes);
Dimension d = ImageSize.getDimensions(fileName,mimeType,inputDim);
// IOUtils.closeQuietly(inputDim);
int newWidth=ImageSize.getResizedWidth(d,maxWidth,maxHeight);
int newHeight=ImageSize.getResizedHeight(d,maxWidth,maxHeight);
ByteArrayInputStream input=new ByteArrayInputStream(bytes);
try {
BufferedImage buffer=createImage(input);
BufferedImage result=scaleImage(buffer,newWidth,newHeight);
return createImage(fileName, mimeType, userId, result);
}finally{
input.close();
}
}catch (ImageSizeException e) {
throw new DaoManagerException("FileName:"+fileName, e);
}catch (FormatNotSuported e) {
throw new DaoManagerException("FileName:"+fileName, e);
}
}
}
public static byte[] createImage(String fileName,String mimeType,byte bytes[],int maxWidth,int maxHeight)throws DaoManagerException,IOException{
if (!ImageSize.isImage(mimeType)){
return null;
} else {
try {
ByteArrayInputStream inputDim=new ByteArrayInputStream(bytes);
Dimension d = ImageSize.getDimensions("memoryFile",mimeType,inputDim);
int newWidth=ImageSize.getResizedWidth(d,maxWidth,maxHeight);
int newHeight=ImageSize.getResizedHeight(d,maxWidth,maxHeight);
ByteArrayInputStream input=new ByteArrayInputStream(bytes);
try {
BufferedImage buffer=createImage(input);
BufferedImage result=scaleImage(buffer,newWidth,newHeight);
ByteArrayOutputStream out=new ByteArrayOutputStream();
ImageUtils.write(mimeType, result,out);
return out.toByteArray();
}finally{
IOUtils.closeQuietly(input);
IOUtils.closeQuietly(inputDim);
}
}catch (ImageSizeException e) {
throw new DaoManagerException("mimeType:"+mimeType, e);
}catch (FormatNotSuported e) {
throw new DaoManagerException("mimeType:"+mimeType, e);
}
}
}
// /**
// */
// public static void rotate90CCw(FileId image)throws DaoManagerException,IOException{
// if (ImageSize.isImage(image)){
// BufferedImage buffer=createImage(image);
// BufferedImage result=rotate90CCw(buffer);
// writeImage(image,result);
// }
// System.gc();
// }
// /**
// */
// public static void rotate90Cw(FileId image)throws DaoManagerException,IOException{
// if (ImageSize.isImage(image)){
// BufferedImage buffer=createImage(image);
// BufferedImage result=rotate90Cw(buffer);
// writeImage(image,result);
// }
// System.gc();
// }
static private BufferedImage rotate90CCw(BufferedImage bi) {
int width = bi.getWidth();
int height = bi.getHeight();
int destWidth = bi.getHeight();
int destHeight = bi.getWidth();
ColorModel cm=bi.getColorModel();
BufferedImage destBi;
if (cm instanceof IndexColorModel){
destBi = new BufferedImage(destWidth, destHeight, bi.getType(),(IndexColorModel)cm);
Raster source=bi.getData();
WritableRaster dest=destBi.getRaster();
// double array[]=new double[16];
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy