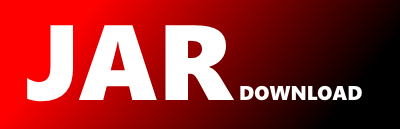
eu.ginere.base.util.lang.MapOfLists Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ginere-base Show documentation
Show all versions of ginere-base Show documentation
Base utilities for java projects
package eu.ginere.base.util.lang;
/**
* Copyright: Angel-Ventura Mendo Gomez
* [email protected]
*
* $Id: MapOfLists.java 21 2007-06-23 00:22:03Z ventura $
*/
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Vector;
import java.util.concurrent.ConcurrentHashMap;
/**
* This is a map of lists. The lists and the maps are syncronized to avoid concurent access
* problems
*
* @author Angel-Ventura Mendo Gomez
* @version $Revision: 21 $ $Date: 2007-06-23 02:22:03 +0200 $
*/
public class MapOfLists {
private final Map> map=new ConcurrentHashMap>();
public MapOfLists(){
}
/**
* This add the element value at the end of the list pointed by key
*/
public void put(K key,V value){
List list;
if (map.containsKey(key)){
list=(List)map.get(key);
} else {
list=new Vector();
map.put(key,list);
}
list.add(value);
}
/**
* This add the element value at the end of the list pointed by key
*/
public List get(K key){
return (List)map.get(key);
}
public boolean containsKey(K key){
return (map.containsKey(key));
}
public void remove(K key){
map.remove(key);
}
/*
public void remove(String key,Object object){
if (containsKey(key)){
List list=get(key);
list.remove(object);
if (list.size()==0){
map.remove(key);
}
}
}
*/
public Collection> values(){
return map.values();
}
public Set keySet(){
return map.keySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy