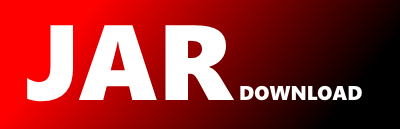
eu.hexagonmc.spigot.gradle.meta.MetadataSpigotExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spigot-gradle Show documentation
Show all versions of spigot-gradle Show documentation
Gradle plugin for Spigot and BungeeCord
The newest version!
/**
*
* Copyright (C) 2017 HexagonMc
* Copyright (C) 2017 Zartec
*
* This file is part of Spigot-Gradle.
*
* Spigot-Gradle is free software:
* you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Spigot-Gradle is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Spigot-Gradle.
* If not, see .
*/
package eu.hexagonmc.spigot.gradle.meta;
import eu.hexagonmc.spigot.annotation.meta.LoadOn;
import eu.hexagonmc.spigot.annotation.meta.PluginMetadata;
import eu.hexagonmc.spigot.gradle.SpigotGradle;
import groovy.lang.Closure;
import org.gradle.api.Project;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class MetadataSpigotExtension extends MetadataExtension {
public static final String EXTENSION_NAME = "spigot";
private Object _load;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy