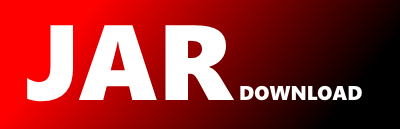
eu.interedition.text.TextStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of text-core Show documentation
Show all versions of text-core Show documentation
Stand-off Markup/Annotation Text Model
The newest version!
package eu.interedition.text;
import com.google.common.base.Throwables;
import java.io.IOException;
/**
* @author Gregor Middell
*/
public interface TextStream {
void stream(Listener listener) throws IOException;
interface Listener {
void start(long contentLength);
void start(long offset, Iterable> layers);
void end(long offset, Iterable> layers);
void text(TextRange r, String text);
void end();
}
/**
* @author Gregor Middell
*/
class ListenerAdapter implements Listener {
public void start(long contentLength) {
}
public void start(long offset, Iterable> layers) {
}
public void end(long offset, Iterable> layers) {
}
public void text(TextRange r, String text) {
}
public void end() {
}
}
/**
* @author Gregor Middell
*/
class ExceptionPropagatingListenerAdapter implements Listener {
public void start(long contentLength) {
try {
doStart(contentLength);
} catch (Exception e) {
throw Throwables.propagate(e);
}
}
public void start(long offset, Iterable> layers) {
try {
doStart(offset, layers);
} catch (Exception e) {
throw Throwables.propagate(e);
}
}
public void end(long offset, Iterable> layers) {
try {
doEnd(offset, layers);
} catch (Exception e) {
throw Throwables.propagate(e);
}
}
public void text(TextRange r, String text) {
try {
doText(r, text);
} catch (Exception e) {
throw Throwables.propagate(e);
}
}
public void end() {
try {
doEnd();
} catch (Exception e) {
throw Throwables.propagate(e);
}
}
protected void doStart(long contentLength) throws Exception {
}
protected void doStart(long offset, Iterable> layers) throws Exception {
}
protected void doEnd(long offset, Iterable> layers) throws Exception {
}
protected void doText(TextRange r, String text) throws Exception {
}
protected void doEnd() throws Exception {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy