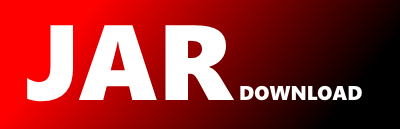
eu.interedition.text.h2.JdbcUtil Maven / Gradle / Ivy
package eu.interedition.text.h2;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.MessageFormat;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* @author Gregor Middell
*/
public class JdbcUtil {
private static final Logger LOGGER = Logger.getLogger(JdbcUtil.class.getName());
public static void closeQuietly(Connection connection) {
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
if (LOGGER.isLoggable(Level.WARNING)) {
LOGGER.log(Level.WARNING, MessageFormat.format("Exception while closing connection {0}", connection), e);
}
}
}
public static void closeQuietly(ResultSet resultSet) {
try {
if (resultSet != null) {
resultSet.close();
}
} catch (SQLException e) {
if (LOGGER.isLoggable(Level.WARNING)) {
LOGGER.log(Level.WARNING, MessageFormat.format("Exception while closing result set {0}", resultSet), e);
}
}
}
public static void closeQuietly(PreparedStatement stmt) {
try {
if (stmt != null) {
stmt.close();
}
} catch (SQLException e) {
if (LOGGER.isLoggable(Level.WARNING)) {
LOGGER.log(Level.WARNING, MessageFormat.format("Exception while closing prepared statement {0}", stmt), e);
}
}
}
public static void closeQuietly(Statement stmt) {
try {
if (stmt != null) {
stmt.close();
}
} catch (SQLException e) {
if (LOGGER.isLoggable(Level.WARNING)) {
LOGGER.log(Level.WARNING, MessageFormat.format("Exception while closing statement {0}", stmt), e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy