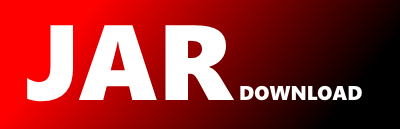
eu.limetri.client.mapviewer.fx.ZoomPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-javafx Show documentation
Show all versions of mapviewer-javafx Show documentation
JXMapViewer JavaFX implementation
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.fx;
import static eu.limetri.client.mapviewer.fx.JFXMapPane.MAX_ZOOM;
import static eu.limetri.client.mapviewer.fx.JFXMapPane.MIN_ZOOM;
import static eu.limetri.client.mapviewer.fx.JFXMapPane.ZOOM;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Orientation;
import javafx.scene.control.Button;
import javafx.scene.control.Slider;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
public class ZoomPanel extends BorderPane {
private static final int BUTTON_SIZE = 20;
private static final ImageView imagePlus = new ImageView(new Image(JFXMapPane.class.getResource("plus.png").toString()));
private static final ImageView imageMinus = new ImageView(new Image(JFXMapPane.class.getResource("minus.png").toString()));
private Button zoomButtonPlus;
private Button zoomButtonMinus;
private Slider zoomSlider;
private JFXMapPane mapPane;
private SimpleIntegerProperty zoomProperty = new SimpleIntegerProperty();
private PropertyChangeListener zoomPropertyChangeListener;
public ZoomPanel(JFXMapPane mapPane) {
super();
this.mapPane = mapPane;
init();
}
private void init() {
zoomButtonMinus = new Button();
zoomButtonPlus = new Button();
zoomButtonMinus.setGraphic(imageMinus);
zoomButtonPlus.setGraphic(imagePlus);
zoomButtonMinus.setMaxSize(BUTTON_SIZE, BUTTON_SIZE);
zoomButtonMinus.setMinSize(BUTTON_SIZE, BUTTON_SIZE);
zoomButtonPlus.setMaxSize(BUTTON_SIZE, BUTTON_SIZE);
zoomButtonPlus.setMinSize(BUTTON_SIZE, BUTTON_SIZE);
zoomSlider = new Slider(MIN_ZOOM, MAX_ZOOM, 0);
zoomSlider.setOrientation(Orientation.VERTICAL);
setCenter(zoomSlider);
setTop(zoomButtonPlus);
setBottom(zoomButtonMinus);
getStyleClass().add("zoom-controls-pane");
zoomButtonPlus.getStyleClass().add("zoom-button");
zoomButtonPlus.getStyleClass().add("plus");
zoomButtonMinus.getStyleClass().add("zoom-button");
zoomButtonMinus.getStyleClass().add("minus");
setMaxWidth(20);
setMaxHeight(150);
zoomSlider.valueProperty().bindBidirectional(zoomProperty);
zoomButtonPlus.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
if (zoomProperty.get() < MAX_ZOOM) {
zoomProperty.set(zoomProperty.get()+1);
}
}
});
zoomButtonMinus.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
if (zoomProperty.get() > MIN_ZOOM) {
zoomProperty.set(zoomProperty.get()-1);
}
}
});
zoomProperty.addListener(new ChangeListener() {
@Override
public void changed(ObservableValue extends Number> observable, Number oldValue, Number newValue) {
setZoom((MAX_ZOOM - zoomProperty.get())+1);
}
});
zoomPropertyChangeListener = new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
setZoomFromMap();
}
};
}
private void setZoomFromMap() {
zoomProperty.set((MAX_ZOOM - mapPane.getZoom())+1);
}
protected void setZoom(int zoom) {
mapPane.setZoom(zoom);
}
public void attach() {
mapPane.addPropertyChangeListener(ZOOM, zoomPropertyChangeListener);
setZoomFromMap();
}
public void detach() {
mapPane.removePropertyChangeListener(ZOOM, zoomPropertyChangeListener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy