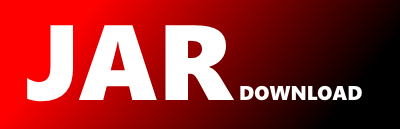
eu.limetri.client.mapviewer.fx.MapMarkerVehicle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-javafx Show documentation
Show all versions of mapviewer-javafx Show documentation
JXMapViewer JavaFX implementation
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.fx;
import eu.limetri.client.mapviewer.fx.api.MapMarker;
import java.awt.Point;
import javafx.scene.Group;
import javafx.scene.effect.DropShadow;
import javafx.scene.effect.Light.Distant;
import javafx.scene.effect.Lighting;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.paint.Color;
/**
*
* @author smithjel
*/
public class MapMarkerVehicle implements MapMarker, Marker {
double lat;
double lon;
double hdg;
ImageView ImgView;
public MapMarkerVehicle(Image img, double lat, double lon, double hdg ) {
super();
this.lat = lat;
this.lon = lon;
this.hdg = hdg;
this.ImgView = new ImageView( img );
Distant light = new Distant();
light.setAzimuth(-135.0f);
Lighting lighting = new Lighting();
lighting.setLight(light);
lighting.setSurfaceScale(5.0f);
// this.ImgView.setEffect(lighting);
DropShadow dropShadow = new DropShadow();
dropShadow.setOffsetX(30);
dropShadow.setOffsetY(30);
dropShadow.setColor(Color.rgb( 0, 0, 0, 0.7));
dropShadow.setInput(lighting);
this.ImgView.setEffect(dropShadow);
}
public double getLat() {
return lat;
}
public void setLat(double val) {
this.lat = val;
}
public double getLon() {
return lon;
}
public void setLon(double val) {
this.lon = val;
}
public double getHdg() {
return hdg;
}
public void setHdg(double val) {
this.hdg = val;
}
public void Render(Group g, Point position) {
int size_w = (int)this.ImgView.getImage().getWidth();
int size_h = (int)this.ImgView.getImage().getHeight();
this.ImgView.setTranslateX(position.x - (size_w / 2));
this.ImgView.setTranslateY(position.y - (size_h / 2));
this.ImgView.setRotate(this.hdg);
g.getChildren().add(this.ImgView);
// g.setColor(color);
// g.fillOval(position.x - size_h, position.y - size_h, size, size);
// g.setColor(Color.BLACK);
// g.drawOval(position.x - size_h, position.y - size_h, size, size);
}
@Override
public String toString() {
return "Vehicle at " + lat + " " + lon;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy