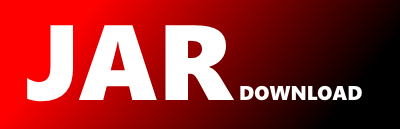
eu.limetri.client.mapviewer.swing.jxmap.map.AbstractLineDrawingContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.swing.jxmap.map;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.CoordinateSequence;
import com.vividsolutions.jts.geom.GeometryFactory;
import com.vividsolutions.jts.geom.LineString;
import com.vividsolutions.jts.geom.impl.CoordinateArraySequence;
import eu.limetri.client.mapviewer.api.SingleObjectLayer;
import eu.limetri.client.mapviewer.data.GeoPosition;
import eu.limetri.client.mapviewer.swing.JXMapViewer;
/**
*
* @author johan
*/
public abstract class AbstractLineDrawingContext extends AbstractDrawingContext {
// FIXME: set WGS-84 SRID or CRS on factory
protected final GeometryFactory geometryFactory = new GeometryFactory();
private SingleObjectLayer layer;
public AbstractLineDrawingContext(SingleObjectLayer layer, JXMapViewer mapViewer) {
super(mapViewer);
this.layer = layer;
}
@Override
public SingleObjectLayer getLayer(){
return this.layer;
}
@Override
protected boolean addPoint(GeoPosition position) {
Coordinate coord = new Coordinate(position.getLongitude(), position.getLatitude());
if (isValidLine(coord)) {
coords.add(coord);
pointAdded();
// notify panel & toolbar actions:
getPropertyChangeSupport().firePropertyChange(PROP_DRAWING_CONTEXT, null, null);
return true;
}
return false;
}
/**
* Check if adding the provided coordinate to the list will produce a valid line.
* The new segment must not intersect any of the existing ones.
*
* @param coord
* @return
*/
protected boolean isValidLine(Coordinate coord) {
int len = coords.size();
// number of points in LineString must be 0 or >= 2)
if (len < 2) {
return true;
}
CoordinateSequence coordSequence1 = new CoordinateArraySequence(coords.toArray(new Coordinate[len]));
CoordinateSequence coordSequence2 = new CoordinateArraySequence(new Coordinate[] {coords.get(len - 1), coord});
LineString lineString1 = new LineString(coordSequence1, geometryFactory);
LineString lineString2 = new LineString(coordSequence2, geometryFactory);
return !lineString1.crosses(lineString2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy