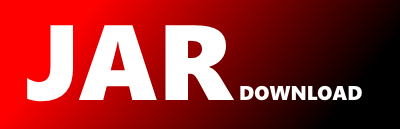
eu.limetri.client.mapviewer.swing.jxmap.map.AreaMeasurementContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.swing.jxmap.map;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.CoordinateSequence;
import com.vividsolutions.jts.geom.GeometryFactory;
import com.vividsolutions.jts.geom.LinearRing;
import com.vividsolutions.jts.geom.Polygon;
import com.vividsolutions.jts.geom.impl.CoordinateArraySequence;
import eu.limetri.client.mapviewer.data.GeoPosition;
import eu.limetri.client.mapviewer.data.util.ScaleUtil;
import eu.limetri.client.mapviewer.swing.JXMapViewer;
import eu.limetri.client.mapviewer.swing.render.DrawingRenderer;
import eu.limetri.client.mapviewer.swing.render.PolygonDrawingRenderer;
import java.awt.BorderLayout;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import eu.agrosense.client.lib.geotools.GeometryTools;
import org.openide.util.NbBundle;
/**
* Context for measuring the area of painted shape
*
* @author Frantisek Post
*/
@NbBundle.Messages({"area_measurement_result_format=Area of the selected shape is: %s",
"area_measurement=Area measurement"})
public class AreaMeasurementContext extends AbstractMeasurementContext {
protected final GeometryFactory geometryFactory = new GeometryFactory();
public AreaMeasurementContext(JXMapViewer mapViewer) {
super(mapViewer);
}
/**
* Create a ring form the currently drawn line by adding a segment from the
* last to the first point.
*
* @return
*/
private LinearRing makeRing() {
int len = coords.size() + 1;
Coordinate[] coordArray = coords.toArray(new Coordinate[len]);
coordArray[len - 1] = coordArray[0]; // close the ring
CoordinateSequence coordSequence = new CoordinateArraySequence(coordArray);
return new LinearRing(coordSequence, geometryFactory);
}
@Override
public boolean canFinish() {
// are there enough points to create a polygon?
if (coords.size() < 3) {
return false;
}
// check if closing the currently drawn line produces a valid ring:
return makeRing().isValid();
}
@Override
public DrawingRenderer getRenderer() {
return new PolygonDrawingRenderer(coords);
}
@Override
public String getDescription() {
return Bundle.area_measurement();
}
@Override
protected JComponent createResultComponent() {
LinearRing ring = makeRing();
Polygon polygon = new Polygon(ring, null, geometryFactory);
double area = GeometryTools.getAreaInHA(polygon);
JPanel panel = new JPanel();
panel.setLayout(new BorderLayout());
JLabel label = new JLabel();
label.setText(String.format(Bundle.area_measurement_result_format(), ScaleUtil.formatArea(area)));
panel.add(label, BorderLayout.CENTER);
panel.setBorder(new EmptyBorder(10, 10, 10, 10));
return panel;
}
@Override
protected void addPointImpl(GeoPosition position) {
Coordinate coord = new Coordinate(position.getLongitude(), position.getLatitude());
coords.add(coord);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy