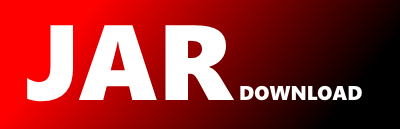
eu.limetri.client.mapviewer.swing.render.DataSetRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/*
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*
* Contributors:
* Timon Veenstra - initial API and implementation and/or initial documentation
*/
package eu.limetri.client.mapviewer.swing.render;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import eu.limetri.api.geo.DataSet;
import eu.limetri.client.mapviewer.api.Palette;
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Paint;
import java.awt.Rectangle;
import java.awt.geom.Point2D;
import org.netbeans.api.visual.model.ObjectState;
/**
*
* @author Timon Veenstra
*/
public class DataSetRenderer {
private static final int POINT_WIDTH_HEIGHT = 10;
/**
* paint the data set
*
* @param dataSet should already be limited to the set within envelope to
* draw
* @param viewport
* @param palette
* @param graphics
* @param state
* @param translator
*/
void paint(DataSet dataSet, Geometry boundingBox, Rectangle viewport, Graphics2D canvas, Palette palette, ObjectState state, GeoTranslator translator) {
canvas.setStroke(new BasicStroke(2, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND));
canvas.setPaint(Color.BLACK);
dataSet.stream().parallel().forEach((record) -> {
Graphics2D g = (Graphics2D)canvas.create();
Coordinate c = new Coordinate(record.getLon(), record.getLat());
final Point2D pointOnMap = translator.geoToPixel(viewport, boundingBox, c);
final int x = (int) (pointOnMap.getX() - viewport.getBounds().getX());
final int y = (int) (pointOnMap.getY() - viewport.getBounds().getY());
g.setPaint(palette.getColorForValue(record.getValue()));
g.fillOval(x, y, POINT_WIDTH_HEIGHT, POINT_WIDTH_HEIGHT);
g.dispose();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy