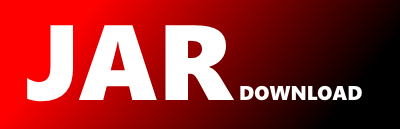
eu.limetri.client.mapviewer.swing.render.GeometricalsPreviewPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.swing.render;
import java.awt.BorderLayout;
import java.awt.Color;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import org.netbeans.api.visual.action.ActionFactory;
import org.netbeans.api.visual.model.ObjectScene;
import org.netbeans.api.visual.model.ObjectSceneEventType;
import org.netbeans.api.visual.model.ObjectSceneListener;
import org.netbeans.api.visual.widget.LayerWidget;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.GeometryCollection;
import eu.limetri.api.geo.Geometrical;
import eu.limetri.client.mapviewer.api.Palette;
/**
* A preview panel for {@link Geometrical} 's
*
* @author Maciek Dulkiewicz
* @author Merijn Zenger
*/
public class GeometricalsPreviewPanel extends JPanel {
private final JScrollPane jScrollPane;
private ObjectScene scene;
private Palette palette;
private static final Color PALETTE_COLOR = Color.RED;
private static final Color BACKGROUND_COLOR = Color.WHITE;
/**
* Create a new GeometricalsPreviewPanel with the provided {@link Geometrical}'s as
* content. The color will be set to {@link GeometricalsPreviewPanel#PALETTE_COLOR}
*
* @param geometricals
*/
public GeometricalsPreviewPanel(List geometricals) {
this(geometricals, new Palette(PALETTE_COLOR));
}
/**
* Create a new GeometricalsPreviewPanel with the provided Geometricals as
* content. The color will be set to the provided palette
*
* @param geometricals
* @param palette
*/
public GeometricalsPreviewPanel(List geometricals, Palette palette) {
this.setLayout(new BorderLayout());
this.setBackground(BACKGROUND_COLOR);
this.palette = palette;
jScrollPane = new javax.swing.JScrollPane();
this.add(jScrollPane);
setGeometricals(geometricals);
}
/**
* Set the palette for the component on this preview panel
*
* @param palette
*/
public void setPalette(Palette palette) {
this.palette = palette;
for (Object object : scene.getObjects()) {
GeometricalWidget geometricalWidget = (GeometricalWidget) scene.findWidget(object);
geometricalWidget.setPalette(palette);
}
}
/**
* Set the {@link Geometrical} for this panel
Removes all the old {@link Geometrical}
* and ads the new ones
*
* @param geometricals
*/
public final void setGeometricals(List geometricals) {
scene = new ObjectScene();
scene.getActions().addAction(ActionFactory.createZoomAction());
scene.getActions().addAction(ActionFactory.createPanAction());
List geometries = new ArrayList();
for (Geometrical geometrical : geometricals) {
if (geometrical.getGeometry() != null) {
geometries.add(geometrical.getGeometry());
}
}
LayerWidget mainLayer = new LayerWidget(scene);
scene.setOpaque(false);
scene.addChild(mainLayer);
if (geometries.size() > 0) {
Geometry[] g = geometries.toArray(new Geometry[geometries.size()]);
GeometryCollection geoCollection = new GeometryCollection(g, g[0].getFactory());
for (Geometrical geometrical : geometricals) {
if (geometrical.getGeometry() != null) {
//The constructor of ShapeWidget requires a geometrical that can be cast to a Shape
GeometricalWidget widget = new GeometricalWidget(scene, new SimpleGeoTranslator(), geometrical, palette, geoCollection.getEnvelope());
widget.getActions().addAction(scene.createSelectAction());
widget.getActions().addAction(scene.createObjectHoverAction());
mainLayer.addChild(widget);
scene.addObject(geometrical, widget);
}
}
}
jScrollPane.setViewportView(scene.createView());
}
/**
* add a listener of type {@link ObjectSceneEventType#OBJECT_SELECTION_CHANGED} on the {@link ObjectScene} of this preview panel
* @param listener
*/
public void addSelectionChangeListener(ObjectSceneListener listener){
scene.addObjectSceneListener(listener, ObjectSceneEventType.OBJECT_SELECTION_CHANGED);
}
/**
* remove a listener of type {@link ObjectSceneEventType#OBJECT_SELECTION_CHANGED} on the {@link ObjectScene} of this preview panel
* @param listener
*/
public void removeSelectionChangeListener(ObjectSceneListener listener){
scene.removeObjectSceneListener(listener, ObjectSceneEventType.OBJECT_SELECTION_CHANGED);
}
/**
* Set the selected Geometrical 's in this preview panel
* @param geometricals
*/
public void setSelectedGeometricals(List geometricals) {
Set notEmptyGeometricals = new HashSet();
for (Geometrical geometrical : geometricals) {
if (geometrical.getGeometry() != null) {
notEmptyGeometricals.add(geometrical);
}
}
scene.setSelectedObjects(notEmptyGeometricals);
repaint();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy