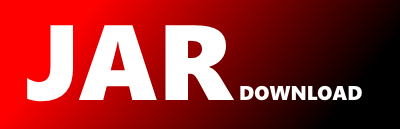
eu.limetri.client.mapviewer.swing.render.GeometryRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.swing.render;
import java.awt.Graphics2D;
import java.awt.Polygon;
import java.awt.Rectangle;
import java.awt.geom.Point2D;
import org.netbeans.api.visual.model.ObjectState;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
/**
* Generic Geometry Renderer
*
* @author Timon Veenstra
* @param
*/
public class GeometryRenderer {
/**
*
* uses ObjectState.createNormal() as rendering state
* final to avoid overriding conflicts.
* Extending classes should only override {@link GeometryRenderer#render(com.vividsolutions.jts.geom.Geometry, com.vividsolutions.jts.geom.Geometry, java.awt.Rectangle, nl.cloudfarming.client.geoviewer.render.GeoTranslator, org.netbeans.api.visual.model.ObjectState, java.awt.Graphics2D) }
*
* @param geometry The geometry to calculate a viewport for
* @param boundingBox collection.getEnvelope(); in case of a geometry collection
* @param canvas mapViewer.getViewportBounds() in case of mapviewer
* @param translator The translator to translate pixels to geo and visa versa
* @param g graphics
*/
public final void render(G geometry, Geometry boundingBox, Rectangle canvas, GeoTranslator translator, Graphics2D g) {
render(geometry, boundingBox, canvas, translator, ObjectState.createNormal(), g);
}
/**
*
* @param geometry The geometry to calculate a viewport for
* @param boundingBox collection.getEnvelope(); in case of a geometry collection
* @param canvas mapViewer.getViewportBounds() in case of mapviewer
* @param translator The translator to translate pixels to geo and visa versa
* @param state Object state, object can be rendered different based on state.
* @param g graphics
*/
public void render(G geometry, Geometry boundingBox, Rectangle canvas, GeoTranslator translator, ObjectState state, Graphics2D g) {
//
// draw the geometry
//
Polygon polygon = new Polygon();
if (geometry.getCoordinates().length > 1) {
for (Coordinate coordinate : geometry.getCoordinates()) {
Point2D point2D = translator.geoToPixel(canvas, boundingBox, coordinate);
int x = (int) (point2D.getX() - canvas.getBounds().getX());
int y = (int) (point2D.getY() - canvas.getBounds().getY());
polygon.addPoint(x, y);
}
g.clip(polygon);
g.fill(polygon);
}
}
/**
* Calculate viewport from specified mapviewer and geometry
*
* @param geometry The geometry to calculate a viewport for
* @param boundingbox collection.getEnvelope(); in case of a geometry collection
* if viewport for single viewport is required pas in bounds of geometry
* @param canvas mapViewer.getViewportBounds() in case of mapviewer
* @param translator The translator to translate pixels to geo and visa versa
* @return
*/
public static Rectangle getViewport(Geometry geometry, Geometry boundingbox, Rectangle canvas, GeoTranslator translator) {
//
// determine the box the geometry will be drawn in
//
Rectangle bounds = new Rectangle(0, 0, -1, -1);
for (Coordinate boundryCoord : geometry.getEnvelope().getCoordinates()) {
Point2D point2D = translator.geoToPixel(canvas, boundingbox, boundryCoord);
int x = (int) (point2D.getX() - canvas.getBounds().getX());
int y = (int) (point2D.getY() - canvas.getBounds().getY());
bounds.add(x, y);
}
return bounds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy