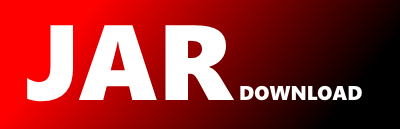
eu.limetri.client.mapviewer.swing.render.PolygonRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mapviewer-swing Show documentation
Show all versions of mapviewer-swing Show documentation
MapViewer Swing implementation
The newest version!
/**
* Copyright (C) 2008-2013 LimeTri. All rights reserved.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package eu.limetri.client.mapviewer.swing.render;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.geom.Point2D;
import java.util.ArrayList;
import java.util.Collection;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.netbeans.api.visual.model.ObjectState;
import com.vividsolutions.jts.awt.PolygonShape;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.Polygon;
import eu.limetri.client.mapviewer.data.GeoPosition;
import java.awt.BasicStroke;
import java.awt.Paint;
import java.awt.TexturePaint;
import java.awt.image.BufferedImage;
/**
* Render a polygon, including holes.
*
* @author johan
*/
public class PolygonRenderer extends GeometryRenderer {
private static final Logger LOGGER = Logger.getLogger(PolygonRenderer.class.getName());
private static final double DEFAULT_DIFFERENCE = 0.0002; //what does this number mean?
private Paint lastPaint;
private Color lastColor;
private int lastSize;
private Paint getPaint(Color color, int size) {
if (lastColor == null || this.lastSize != size || !this.lastColor.equals(color)) {
BufferedImage bi = new BufferedImage(size, size, BufferedImage.TYPE_INT_RGB);
Graphics2D big = bi.createGraphics();
big.setColor(Color.WHITE);
big.fillRect(0, 0, size, size);
big.setColor(color);
big.fillRect(0, 0, size, size);
big.setColor(Color.BLACK);
big.drawLine(0, size - 1, size - 1, 0);
this.lastColor = color;
Rectangle r = new Rectangle(0, 0, size, size);
this.lastPaint = new TexturePaint(bi, r);
this.lastSize = size;
}
return lastPaint;
}
@Override
public void render(Polygon geometry, Geometry boundingBox, Rectangle canvas, GeoTranslator translator, ObjectState state, Graphics2D g) {
Coordinate[] shell = translate(geometry.getExteriorRing().getCoordinates(), boundingBox, canvas, translator);
Collection holes = new ArrayList(geometry.getNumInteriorRing());
for (int i = 0; i < geometry.getNumInteriorRing(); i++) {
holes.add(translate(geometry.getInteriorRingN(i).getCoordinates(), boundingBox, canvas, translator));
}
PolygonShape polygonShape = new PolygonShape(shell, holes);
Color org = g.getColor();
int size = 20;
if (state.isSelected()) {
//
// gradient fill for the selected polygon, makes selection more visible when zoomed out.
//
Point2D edge = new Point2D.Double(polygonShape.getBounds2D().getMinX(), polygonShape.getBounds2D().getMinY());
Point2D centroid = new Point2D.Double(polygonShape.getBounds2D().getCenterX(), polygonShape.getBounds2D().getCenterY());
LOGGER.log(Level.FINEST, "object selected, edge={0}, centroid={1}", new Object[]{edge, centroid});
//
// calculate zoom level and resize factor
//
// - take 2 awt point with a 10 pixel distance.
// - convert those pixels to geo positions
// - calculate distance between the geo positions
// (it's not really the distance but rather the difference in coordinates)
// - the ratio between the distance in pixels and the distance in geo positions
// determines the zoom level. By inverting this number with a max of 1 we get a
// resize factor.
//
//
Point2D left = new java.awt.Point(0, 0);
GeoPosition leftPosition = translator.pixelToGeo(canvas, boundingBox, left);
Point2D slightlyMoreToTheRight = new java.awt.Point(10, 0);
GeoPosition rightPosition = translator.pixelToGeo(canvas, boundingBox, slightlyMoreToTheRight);
final double difference = rightPosition.getLongitude() - leftPosition.getLongitude();
double zoomLevel = difference / DEFAULT_DIFFERENCE;
final double iconResizeFactor = 1.0 / Math.max(zoomLevel /*- 2*/, 1);
if (iconResizeFactor < 0.25f && iconResizeFactor >= 0.1f) {
size = 10;
}
if (iconResizeFactor < 0.1f) {
size = 5;
}
g.setPaint(getPaint(org, size));
}
//
// fill the polygon
//
System.out.println("polygon::" + polygonShape.getBounds().toString());
g.fill(polygonShape);
g.setPaint(org);
if (state.isSelected()) {
final float dash1[] = {size / 2};
BasicStroke dashed = new BasicStroke(1.0f, BasicStroke.CAP_BUTT, BasicStroke.JOIN_MITER, size / 2, dash1, 0.0f);
g.setStroke(dashed);
g.setColor(Color.WHITE);
g.draw(polygonShape);
g.setColor(org);
}
}
private Coordinate[] translate(Coordinate[] coordinates, Geometry boundingBox, Rectangle canvas, GeoTranslator translator) {
Coordinate[] translated = new Coordinate[coordinates.length];
for (int i = 0; i < coordinates.length; i++) {
System.out.println("polygon::" + coordinates[i].toString());
Point2D point2D = translator.geoToPixel(canvas, boundingBox, coordinates[i]);
double x = point2D.getX() - canvas.getBounds().getX();
double y = point2D.getY() - canvas.getBounds().getY();
// truncate to int to prevent blurry boundary (see limetri/agrosense #114)
translated[i] = new Coordinate((int) x, (int) y);
}
return translated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy